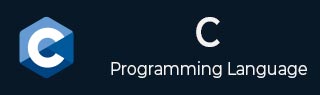
- The C Standard Library
- C Library - Home
- C Library - <assert.h>
- C Library - <complex.h>
- C Library - <ctype.h>
- C Library - <errno.h>
- C Library - <fenv.h>
- C Library - <float.h>
- C Library - <inttypes.h>
- C Library - <iso646.h>
- C Library - <limits.h>
- C Library - <locale.h>
- C Library - <math.h>
- C Library - <setjmp.h>
- C Library - <signal.h>
- C Library - <stdalign.h>
- C Library - <stdarg.h>
- C Library - <stdbool.h>
- C Library - <stddef.h>
- C Library - <stdio.h>
- C Library - <stdlib.h>
- C Library - <string.h>
- C Library - <tgmath.h>
- C Library - <time.h>
- C Library - <wctype.h>
- C Standard Library Resources
- C Library - Quick Guide
- C Library - Useful Resources
- C Library - Discussion
- C Programming Resources
- C Programming - Tutorial
- C - Useful Resources
C library - casinh() function
The C complex library casinh() function is used to calculate the complex arc hyperbolic sine, i.e. inverse hyperbolic sine of z with branch cuts that is lie outside the interval [−i,+i] along the imaginary axis. It makes the function continuous and single-valued for any complex number z not lying in this interval on the imaginary axis.
The inverse hyperbolic sine (asinh) z is defined as: asinh(z)=ln(z + √(z2 + 1))
This function is depends on the type of z(complex number). If the z is the "float" type, we use casinhf() to compute asinh, For long double type, use casinhl(), and for double type, use casinh().
Syntax
Following is the C library syntax of casinh() function −
double complex casinh( double complex z );
Parameters
This function accepts a single parameter −
-
Z − It represent a complex number for which we want to calculate asinh.
Return Value
This function returns the complex arc hyperbolic sine of z, the values are unbounded, meaning they can be any real number. Along the imaginary axis, in the interval [−iπ/2,+iπ/2].
Example 1
Following is the basic c program to demonstrate the use of casinh() on a complex number.
#include <stdio.h> #include <complex.h> int main() { double complex z = 3.0 + 4.0 * I; // Calculate the asinh double complex res = casinh(z); printf("Complex sinh: %.2f%+.2fi\n", creal(res), cimag(res)); return 0; }
Output
Following is the output −
Complex sinh: 2.30+0.92i
Example 2
Let's see another example, calculate the arc hyperbolic sine of real axis using casinh() function.
#include <stdio.h> #include <math.h> #include <complex.h> int main(void) { // real axis double complex z = casinh(1); printf("asinh(1+0i) = %f+%fi \n", creal(z), cimag(z)); }
Output
Following is the output −
asinh(1+0i) = 0.881374+0.000000i
Example 3
The below program, calculate the arc hyperbolic sine of the imaginary axis using casinh() function.
#include <stdio.h> #include <math.h> #include <complex.h> int main(void) { // imaginary axis double complex z2 = casinh(I); printf("asinh(0+1i) = %f+%fi \n", creal(z2), cimag(z2)); }
Output
Following is the output −
asinh(0+1i) = 0.000000+1.570796i