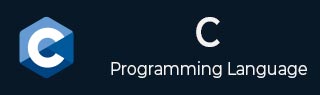
- The C Standard Library
- C Library - Home
- C Library - <assert.h>
- C Library - <complex.h>
- C Library - <ctype.h>
- C Library - <errno.h>
- C Library - <fenv.h>
- C Library - <float.h>
- C Library - <inttypes.h>
- C Library - <iso646.h>
- C Library - <limits.h>
- C Library - <locale.h>
- C Library - <math.h>
- C Library - <setjmp.h>
- C Library - <signal.h>
- C Library - <stdalign.h>
- C Library - <stdarg.h>
- C Library - <stdbool.h>
- C Library - <stddef.h>
- C Library - <stdio.h>
- C Library - <stdlib.h>
- C Library - <string.h>
- C Library - <tgmath.h>
- C Library - <time.h>
- C Library - <wctype.h>
- C Standard Library Resources
- C Library - Quick Guide
- C Library - Useful Resources
- C Library - Discussion
- C Programming Resources
- C Programming - Tutorial
- C - Useful Resources
C library - casin() function
The C complex library casin() function operates the complex arcsine of a given complex number. This function is available in C99 and works with complex numbers. Its behavior is completely different from asin(). A asin() function implement the inverse of sine of a number(in radian).
Syntax
Following is the C library syntax of the casin() function −
double complex ccasin(double complex z);
Parameters
It accept only a single parameter z(complex number) which performs the task of arcsine.
Return Value
When no error occurs, the function returns the complex arcsine of z.
Example 1
Following is the C library program that shows the usage of casin() function.
#include <stdio.h> #include <complex.h> #include <math.h> int main() { double complex z = 1.0 + 2.0 * I; double complex result = casin(z); printf("casin(%lf + %lfi) = %lf + %lfi\n", creal(z), cimag(z), creal(result), cimag(result)); return 0; }
Output
On execution of above code, we get the following result −
casin(1.000000 + 2.000000i) = 0.427079 + 1.528571i
Example 2
Here, we demonstrate the series formula of casin to find the number of terms using recursion and display the result with the help of some pre-existing functions such as creal(), cimag(), and cimag().
#include <stdio.h> #include <complex.h> double complex casin_recursive(double complex z, int n) { if (n == 0) { return z; } double complex term = -(z * z) * (2 * n - 1) / (2 * n) * casin_recursive(z, n - 1); return term; } int main() { double complex z = 1.0 + 2.0 * I; int terms = 10; double complex result = casin_recursive(z, terms); printf("casin(%lf + %lfi) = %lf + %lfi (approximated with %d terms)\n", creal(z), cimag(z), creal(result), cimag(result), terms); return 0; }
Output
After executing the above code, we get the following result −
` casin(1.000000 + 2.000000i) = -1180400.258221 + -3662001.649712i (approximated with 10 terms)