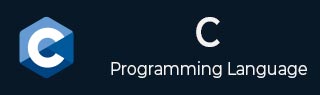
- The C Standard Library
- C Library - Home
- C Library - <assert.h>
- C Library - <complex.h>
- C Library - <ctype.h>
- C Library - <errno.h>
- C Library - <fenv.h>
- C Library - <float.h>
- C Library - <inttypes.h>
- C Library - <iso646.h>
- C Library - <limits.h>
- C Library - <locale.h>
- C Library - <math.h>
- C Library - <setjmp.h>
- C Library - <signal.h>
- C Library - <stdalign.h>
- C Library - <stdarg.h>
- C Library - <stdbool.h>
- C Library - <stddef.h>
- C Library - <stdio.h>
- C Library - <stdlib.h>
- C Library - <string.h>
- C Library - <tgmath.h>
- C Library - <time.h>
- C Library - <wctype.h>
- C Standard Library Resources
- C Library - Quick Guide
- C Library - Useful Resources
- C Library - Discussion
- C Programming Resources
- C Programming - Tutorial
- C - Useful Resources
C library - cacosh() function
The C complex library cacosh() function is used to calculate the complex arc hyperbolic cosine, i.e. inverse hyperbolic cosine of z with branch cut at value less than 1 along with real axis. This means that the function is continuous and single-valued everywhere except along the real axis where z<1.
The inverse hyperbolic cosine (acosh) z is defined as: acosh(z)=ln(z + √(z2 - 1))
This function is depends on the type of z. If the z is the "float" type, we use cacoshf() to compute acosh, For long double type, use cacoshl(), and for double type, use cacosh().
Syntax
Following is the C library syntax of cacosh() function −
double complex cacosh( double complex z );
Parameters
This function accepts a single parameter −
-
Z − It represent a complex number for which we want to calculate acosh.
Return Value
This function returns the complex inverse hyperbolic cosine of z in the interval [0; ∞) along the real axis and in the interval [−iπ +iπ] along the imaginary axis.
Example 1
Following is the basic c program to demonstrate the use of cacosh() on a complex number.
#include <stdio.h> #include <complex.h> int main() { double complex z = 2.0 + 3.0 * I; // Calculate the hyperbolic cosine double complex res = cacosh(z); printf("Inverse hyperbolic cosine: %.2f%+.2fi\n", creal(res), cimag(res)); return 0; }
Output
Following is the output −
Inverse hyperbolic cosine: 1.98+1.00i
Example 2
Let's see another example, calculate the inverse hyperbolic cosine of real axis using cacosh() function.
#include <stdio.h> #include <math.h> #include <complex.h> int main(void) { // inverse cosine of real axis double complex z = 1; double complex res = cacosh(z); printf("acosh(1+0i) = %f+%fi \n", creal(res), cimag(res)); }
Output
Following is the output −
acosh(1+0i) = 0.000000+0.000000i
Example 3
The below C program, calculates the complex arc hyperbolic cosine after computing the conjugate of a complex number.
#include <stdio.h> #include <complex.h> #include <math.h> int main() { double complex z = 1.0 + 2.0 * I; // Calculate the conjugate of z double complex conjugate = conj(z); // Compute the acosh of the conjugate double complex result = acosh(conjugate); // Display the result printf("Conjugate of z: %.2f + %.2fi\n", creal(conjugate), cimag(conjugate)); printf("acosh(conjugate): %.2f + %.2fi\n", creal(result), cimag(result)); return 0; }
Output
Following is the output −
Conjugate of z: 1.00 + -2.00i acosh(conjugate): 0.00 + 0.00i