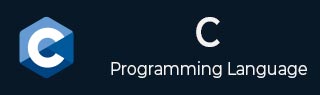
- The C Standard Library
- C Library - Home
- C Library - <assert.h>
- C Library - <complex.h>
- C Library - <ctype.h>
- C Library - <errno.h>
- C Library - <fenv.h>
- C Library - <float.h>
- C Library - <inttypes.h>
- C Library - <iso646.h>
- C Library - <limits.h>
- C Library - <locale.h>
- C Library - <math.h>
- C Library - <setjmp.h>
- C Library - <signal.h>
- C Library - <stdalign.h>
- C Library - <stdarg.h>
- C Library - <stdbool.h>
- C Library - <stddef.h>
- C Library - <stdio.h>
- C Library - <stdlib.h>
- C Library - <string.h>
- C Library - <tgmath.h>
- C Library - <time.h>
- C Library - <wctype.h>
- C Standard Library Resources
- C Library - Quick Guide
- C Library - Useful Resources
- C Library - Discussion
- C Programming Resources
- C Programming - Tutorial
- C - Useful Resources
C library - cacos() function
The C complex library cacos() function perform the task of complex arcsine or inverse cosine of a given complex number. This function is defined under the header <complex.h> and it is available since C99.
Syntax
Following is the C library syntax of the cacos() function −
double complex cacos(double complex z);
Parameters
This function accept only a single parameter −
- z: It is a complex number which we want to operate the arcsine.
Return Value
The function returns the complex arcsine value(z), when no error occurs.
Example 1
Following is the C library program that shows the usage of cacos() function.
#include <stdio.h> #include <complex.h> #include <math.h> int main() { double complex z = 1.0 + 2.0 * I; double complex result = cacos(z); printf("cacos(%lf + %lfi) = %lf + %lfi\n", creal(z), cimag(z), creal(result), cimag(result)); return 0; }
Output
On execution of above code, we get the following result −
cacos(1.000000 + 2.000000i) = 1.143718 + -1.528571i
Example 2
Below the program implement the series formula term = -(z * z) * (2 * i - 1) / (2 * i) using loop iterators within the recursive function.
#include <stdio.h> #include <complex.h> double complex cacos_sol(double complex z, int n) { double complex sum = z; double complex term = z; for (int i = 1; i <= n; ++i) { term *= -(z * z) * (2 * i - 1) / (2 * i); sum += term; } return sum; } int main() { double complex z = 1.0 + 2.0 * I; int terms = 10; double complex result = cacos_sol(z, terms); printf("cacos(%lf + %lfi) = %lf + %lfi (approximated with %d terms)\n", creal(z), cimag(z), creal(result), cimag(result), terms); return 0; }
Output
After executing the above code, we get the following result −
cacos(1.000000 + 2.000000i) = -522414.418148 + -4291552.656029i (approximated with 10 terms)
Example 3
The custom function cacos_recursive() accepts two parameters which is z and n to calculate the number of terms. When the nth terms reaches to 0, the function returns the result value as z(complex number).
#include <stdio.h> #include <complex.h> double complex cacos_recursive(double complex z, int n) { if (n == 0) { return z; } double complex term = -(z * z) * (2 * n - 1) / (2 * n) * cacos_recursive(z, n - 1); return term; } int main() { double complex z = 1.0 + 2.0 * I; int terms = 10; double complex result = cacos_recursive(z, terms); printf("cacos(%lf + %lfi) = %lf + %lfi (approximated with %d terms)\n", creal(z), cimag(z), creal(result), cimag(result), terms); return 0; }
Output
The above code produces the following result −
cacos(1.000000 + 2.000000i) = -522414.418148 + -4291552.656029i (approximated with 10 terms)