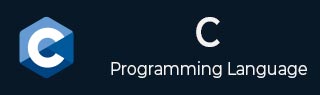
- The C Standard Library
- C Library - Home
- C Library - <assert.h>
- C Library - <complex.h>
- C Library - <ctype.h>
- C Library - <errno.h>
- C Library - <fenv.h>
- C Library - <float.h>
- C Library - <inttypes.h>
- C Library - <iso646.h>
- C Library - <limits.h>
- C Library - <locale.h>
- C Library - <math.h>
- C Library - <setjmp.h>
- C Library - <signal.h>
- C Library - <stdalign.h>
- C Library - <stdarg.h>
- C Library - <stdbool.h>
- C Library - <stddef.h>
- C Library - <stdio.h>
- C Library - <stdlib.h>
- C Library - <string.h>
- C Library - <tgmath.h>
- C Library - <time.h>
- C Library - <wctype.h>
- C Standard Library Resources
- C Library - Quick Guide
- C Library - Useful Resources
- C Library - Discussion
- C Programming Resources
- C Programming - Tutorial
- C - Useful Resources
C library - cabs() function
The C complex library cabs() function is used to obtain the complex absolute value (it is also known as norms, modulus, or magnitude) of z.
This function is depends on the type of z(complex number). If the z is of the "float" type or float imaginary, we can use cabsf() to get absolute value, For type long double, use cabsl(), and for type double, use cabs().
Syntax
Following is the C library syntax of cabs() function −
double cabs( double complex z );
Parameters
This function accepts a single parameter −
-
Z − It represent a complex number for which we want to compute the absolute value.
Return Value
This function returns the absolute value (norm, magnitude) of z if no errors occur.
Example 1
Following is the basic c program to demonstrate the use of cabs() to obtain absolute value of the complex number(z).
#include <stdio.h> #include <complex.h> int main(void) { double complex z = 3.5 + 2.21 * I; double result = cabs(z); printf("cabs(%.2f + %.2fi) = %.3f\n", creal(z), cimag(z), result); return 0; }
Output
Following is the output −
cabs(3.50 + 2.21i) = 4.139
Example 2
Let's see another example, we create a complex number using the CMPLX(). Then we use cabs() function to obtain the absolute value of complex number.
#include <stdio.h> #include <complex.h> int main() { double real = 5.0; double imag = 6.0; // Use the CMPLX function to create complex number double complex z = CMPLX(real, imag); printf("The complex number is: %.2f + %.2fi\n", creal(z), cabs(z)); // use cabs() double absolute_val = cabs(z); printf("The magnitude of z: %.2f\n", absolute_val); return 0; }
Output
Following is the output −
The complex number is: 5.00 + 7.81i The magnitude of z: 7.81
Example 3
The below example use the cabs() function to calculate the absolute value of the resultant complex number.
#include <stdio.h> #include <complex.h> int main() { long double complex z1 = 3.0 + 5.0 * I; long double complex z2 = 4.0 + 3.0 * I; // Difference of two complex numbers long double complex z = z2 - z1; printf("z: %.1Lf + %.1Lfi\n", creall(z), cimagl(z)); printf("Complex Absolute value: %.2Lf\n", cabsl(z)); return 0; }
Output
Following is the output −
z: 1.0 + -2.0i Complex Absolute value: 2.24