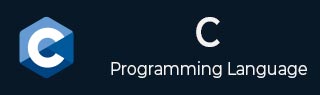
- The C Standard Library
- C Library - Home
- C Library - <assert.h>
- C Library - <complex.h>
- C Library - <ctype.h>
- C Library - <errno.h>
- C Library - <fenv.h>
- C Library - <float.h>
- C Library - <inttypes.h>
- C Library - <iso646.h>
- C Library - <limits.h>
- C Library - <locale.h>
- C Library - <math.h>
- C Library - <setjmp.h>
- C Library - <signal.h>
- C Library - <stdalign.h>
- C Library - <stdarg.h>
- C Library - <stdbool.h>
- C Library - <stddef.h>
- C Library - <stdio.h>
- C Library - <stdlib.h>
- C Library - <string.h>
- C Library - <tgmath.h>
- C Library - <time.h>
- C Library - <wctype.h>
- C Standard Library Resources
- C Library - Quick Guide
- C Library - Useful Resources
- C Library - Discussion
- C Programming Resources
- C Programming - Tutorial
- C - Useful Resources
C library - bsearch() function
The C stdlib library bsearch() function performs a binary search on a sorted array. It is used to find a specific element within the array. This function searches through an array of n objects pointed to by base. It looks for an element matching the object pointed to by key.
A binary search is a searching algorithm used to find the position of the targeted value within the sorted array.
Syntax
Following is the C library syntax of the bsearch() function −
void *bsearch(const void *key, const void *base, size_t nitems, size_t size, int (*compar)(const void *, const void *))
Parameters
This function accepts following parameters −
key − It represents pointer to the element that need to be search.
base − It represents pointer to the first element of the array.
nitems − It represents number of element in the array.
size − It represents size of each element in the array.
compare − It represent a function that compares two elements.
Return Value
This function returns a pointer to the matched element in the array. Otherwise, it returns NULL pointer.
Example 1
In this example, we create a basic c program to demonstrate the use of bsearch() function.
#include <stdio.h> #include <stdlib.h> int compare(const void * a, const void * b) { return ( *(int*)a - *(int*)b ); } // main function int main () { int values[] = { 5, 20, 10, 30, 40 }; int *item; int key = 30; /* using bsearch() to find value 30 in the array */ item = (int*) bsearch (&key, values, 5, sizeof (int), compare); if( item != NULL ) { printf("Found item = %d\n", *item); } else { printf("Item = %d could not be found\n", *item); } return(0); }
Output
Following is the output −
Found item = 30
Example 2
The below c program uses the bsearch() function to search for a character in a sorted array.
#include <stdio.h> #include <stdlib.h> // Comparison function int comfunc(const void* a, const void* b) { return (*(char*)a - *(char*)b); } // main function int main() { // sorted array char arr[] = {'a', 'b', 'c', 'd', 'e'}; int n = sizeof(arr) / sizeof(arr[0]); // searching key char key = 'c'; // using bsearch() char* item = (char*)bsearch(&key, arr, n, sizeof(char), comfunc); // If the key is found, print its value and index if (item != NULL) { printf("'%c' Found at index %ld\n", *item, item - arr); } else { printf("Character '%c' is not found\n", key); } return 0; }
Output
Following is the output −
'c' Found at index 2
Example 3
Following is the another example, we create a c program to use the bsearch() function to search for a string in a sorted array.
#include <stdio.h> #include <stdlib.h> #include <string.h> // Comparison function int comfunc(const void* a, const void* b) { return strcmp(*(const char**)a, *(const char**)b); } // main function int main() { // Sorted array of strings const char* arr[] = {"Aman", "Shriansh", "Tapas", "Vivek"}; int n = sizeof(arr) / sizeof(arr[0]); // Searching key const char* key = "Aman"; // Using bsearch() const char** item; item = (const char**)bsearch(&key, arr, n, sizeof(const char*), comfunc); // If the key is found, print its value and index if (item != NULL) { printf("'%s' Found at index %ld\n", *item, item - arr); } else { printf("Name '%s' is not found\n", key); } return 0; }
Output
Following is the output −
'Aman' Found at index 0