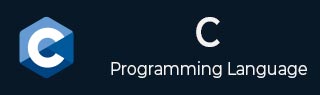
- The C Standard Library
- C Library - Home
- C Library - <assert.h>
- C Library - <complex.h>
- C Library - <ctype.h>
- C Library - <errno.h>
- C Library - <fenv.h>
- C Library - <float.h>
- C Library - <inttypes.h>
- C Library - <iso646.h>
- C Library - <limits.h>
- C Library - <locale.h>
- C Library - <math.h>
- C Library - <setjmp.h>
- C Library - <signal.h>
- C Library - <stdalign.h>
- C Library - <stdarg.h>
- C Library - <stdbool.h>
- C Library - <stddef.h>
- C Library - <stdio.h>
- C Library - <stdlib.h>
- C Library - <string.h>
- C Library - <tgmath.h>
- C Library - <time.h>
- C Library - <wctype.h>
- C Standard Library Resources
- C Library - Quick Guide
- C Library - Useful Resources
- C Library - Discussion
- C Programming Resources
- C Programming - Tutorial
- C - Useful Resources
C library - atan2() function
The C math library atan2() function is used to returns the arctangent in radians of y/x based on the signs of both values to determine the correct quadrant.
The arctangent, also known as the Inverse tangent. It is the inverse of the tangent function, which reverses the tangent function's effect.
The angle returned by this method is measured counter-clockwise from the positive x-axis to the point.
Syntax
Following is the syntax of the C atan2() function −
double atan2( double y, double x );
Parameters
This function accepts following parameters −
-
x − It represent x-coordinate of floating point type.
-
Y − It represent y-coordinate of floating point type.
Return Value
This function returns the angle Θ (in radians) from the conversion of rectangular coordinates (, ) to polar coordinates (, Θ).
Example 1
Following is the basic c program to demonstrate the use of atan2() to obtain an angle in radians.
#include <stdio.h> #include <math.h> int main() { double x = 1.0; double y = 1.0; double theta = atan2(y, x); printf("The angle is %f radians.\n", theta); return 0; }
Output
Following is the output −
The angle is 0.785398 radians.
Example 2
Let's create another example, the point (x,y) = (1,1) is in the second quadrant. The atan2() function calculates the angle formed with the positive x-axis.
#include <stdio.h> #include <math.h> int main() { double x = -1.0; double y = 1.0; double theta = atan2(y, x); printf("The angle is %f radians.\n", theta); return 0; }
Output
Following is the output −
The angle is 2.356194 radians.
Example 3
Now, Create another c program to display the value of the arctangent in the degree.
#include <stdio.h> #include <math.h> #define PI 3.14159265 int main () { double x, y, ang_res, val; x = 10.0; y = 10.0; val = 180.0 / PI; ang_res = atan2 (y,x) * val; printf("The arc tangent of x = %lf, y = %lf ", x, y); printf("is %lf degrees\n", ang_res); return(0); }
Output
Following is the output −
The arc tangent of x = 10.000000, y = 10.000000 is 45.000000 degrees