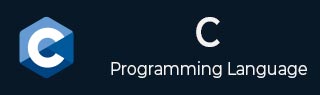
- The C Standard Library
- C Library - Home
- C Library - <assert.h>
- C Library - <complex.h>
- C Library - <ctype.h>
- C Library - <errno.h>
- C Library - <fenv.h>
- C Library - <float.h>
- C Library - <inttypes.h>
- C Library - <iso646.h>
- C Library - <limits.h>
- C Library - <locale.h>
- C Library - <math.h>
- C Library - <setjmp.h>
- C Library - <signal.h>
- C Library - <stdalign.h>
- C Library - <stdarg.h>
- C Library - <stdbool.h>
- C Library - <stddef.h>
- C Library - <stdio.h>
- C Library - <stdlib.h>
- C Library - <string.h>
- C Library - <tgmath.h>
- C Library - <time.h>
- C Library - <wctype.h>
- C Standard Library Resources
- C Library - Quick Guide
- C Library - Useful Resources
- C Library - Discussion
- C Programming Resources
- C Programming - Tutorial
- C - Useful Resources
C library - atan() function
The C math library atan() function is used to returns the arc tangent (inverse tangent) of passed 'arg' in radians in the range [-π/2, +π/2].
The inverse tangent, also known as the arctangent. It is the inverse of the tangent function, which reverses the tangent function's effect.
Syntax
Following is the syntax of the C atan() function −
double atan( double arg );
Parameters
This function accepts a single parameter −
-
arg − It represent a floating point number.
Return Value
If no errors occur, the arc tangent of the argument (arg) is returned in the range [-π/2, +π/2] in radians. If a range error occurs due to underflow, the correct result (after rounding) is returned.
Example 1
Following is the basic c program to demonstrate the use of atan() to obtain an angle in radians.
#include <stdio.h> #include <math.h> int main() { double arg = 1.0; double res = atan(arg); printf("The arc tangent of %f is %f radians.\n", arg, res); return 0; }
Output
Following is the output −
The arc tangent of 1.000000 is 0.785398 radians.
Example 2
Let's create another example, we use the atan() function to obtain the inverse tangent value of the each element of the array.
#include <stdio.h> #include <math.h> int main() { double arg[] = {10, 20, 25, 30}; int length = sizeof(arg) / sizeof(arg[0]); for(int i=0; i<length; i++){ double res = atan(arg[i]); printf("The arc tangent of %f is %f radians.\n", arg[i], res); } return 0; }
Output
Following is the output −
The arc tangent of 10.000000 is 1.471128 radians. The arc tangent of 20.000000 is 1.520838 radians. The arc tangent of 25.000000 is 1.530818 radians. The arc tangent of 30.000000 is 1.537475 radians.
Example 3
Now, Create another c program to display the value of the arctangent in the degree.
#include <stdio.h> #include <math.h> #define PI 3.14159265 int main () { double arg, res_in_degree, val; arg = 1.0; val = 180.0 / PI; res_in_degree = atan (arg) * val; printf("The arc tangent of %lf is %lf degrees", arg, res_in_degree); return(0); }
Output
Following is the output −
The arc tangent of 1.000000 is 45.000000 degrees