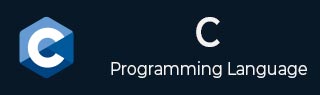
- The C Standard Library
- C Library - Home
- C Library - <assert.h>
- C Library - <complex.h>
- C Library - <ctype.h>
- C Library - <errno.h>
- C Library - <fenv.h>
- C Library - <float.h>
- C Library - <inttypes.h>
- C Library - <iso646.h>
- C Library - <limits.h>
- C Library - <locale.h>
- C Library - <math.h>
- C Library - <setjmp.h>
- C Library - <signal.h>
- C Library - <stdalign.h>
- C Library - <stdarg.h>
- C Library - <stdbool.h>
- C Library - <stddef.h>
- C Library - <stdio.h>
- C Library - <stdlib.h>
- C Library - <string.h>
- C Library - <tgmath.h>
- C Library - <time.h>
- C Library - <wctype.h>
- C Standard Library Resources
- C Library - Quick Guide
- C Library - Useful Resources
- C Library - Discussion
- C Programming Resources
- C Programming - Tutorial
- C - Useful Resources
C library - CMPLX() function
The C complex library CMPLX() function is typically used to generate a complex number composed by its real and imaginary (imag) parts.
If the real and imaginary parts are of the "float" type, we can use cmplxf() to generate complex numbers and crealf() and cimagf() to get the real and imaginary parts, and For long double, use cmplxl(), creall(), and cimagl().
This function may be used for the scientific and engineering application, including signal processing, control system, and quantum mechanics.
Syntax
Following is the C library syntax of CMPLX() function −
double complex CMPLX( double real, double imag );
Parameters
This function accepts following parameters −
-
real − It represent the real part of the complex number. It is of double type.
-
imag − It represent the imaginary (imag) part of the complex number. It is of double type.
Return Value
This function returns a complex number of type double.
Example 1
Following is the basic c program to demonstrate the use of CMPLX() to generate the complex number.
#include <stdio.h> #include <complex.h> int main() { double real = 3.0; double imag = 4.0; // Use the CMPLX function to create complex number double complex z = CMPLX(real, imag); printf("The complex number is: %.2f + %.2fi\n", creal(z), cimag(z)); return 0; }
Output
Following is the output −
The complex number is: 3.00 + 4.00i
Example 2
Calculate Conjugate
Let's see another example, we use the CMPLX() function to create complex number, then we used 'conj()' to calculate the conjugate of a complex number.
#include <stdio.h> #include <complex.h> int main() { double real = 3.0; double imag = 4.0; // Use the CMPLX function to create complex number double complex z = CMPLX(real, imag); printf("The complex number is: %.2f + %.2fi\n", creal(z), cimag(z)); // Calculate conjugate double complex z_conjugate = conj(z); printf("The conjugate of the complex number is: %.2f + %.2fi\n", creal(z_conjugate), cimag(z_conjugate)); return 0; }
Output
Following is the output −
The complex number is: 3.00 + 4.00i The conjugate of the complex number is: 3.00 + -4.00i
Example 3
Perform Arithmetic operation on complex number
The below example generate two complex number and perform the arithmetic operation on these two.
#include <stdio.h> #include <complex.h> int main() { double real1 = 2.0, imag1 = 3.0; double real2 = 4.0, imag2 = -5.0; // Use the CMPLX function double complex z1 = CMPLX(real1, imag1); double complex z2 = CMPLX(real2, imag2); // Arithmetic operations double complex sum = z1 + z2; double complex difference = z1 - z2; // Display the results of the operations printf("Complex number z1: %.2f + %.2fi\n", creal(z1), cimag(z1)); printf("Complex number z2: %.2f + %.2fi\n", creal(z2), cimag(z2)); printf("Sum: %.2f + %.2fi\n", creal(sum), cimag(sum)); printf("Difference: %.2f + %.2fi\n", creal(difference), cimag(difference)); return 0; }
Output
Following is the output −
Complex number z1: 2.00 + 3.00i Complex number z2: 4.00 + -5.00i Sum: 6.00 + -2.00i Difference: -2.00 + 8.00i