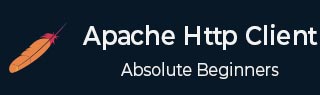
- Apache HttpClient Tutorial
- Home
- Overview
- Environment Setup
- Http Get Request
- Http Post Request
- Response Handlers
- Closing Connection
- Aborting a Request
- Interceptors
- User Authentication
- Using Proxy
- Proxy Authentication
- Form-Based Login
- Cookies Management
- Multiple Threads
- Custom SSL Context
- Multipart Upload
- Apache HttpClient Resources
- Quick Guide
- Useful Resources
- Discussion
Apache HttpClient - Response Handlers
Processing the HTTP responses using the response handlers is recommended. In this chapter, we are going to discuss how to create response handlers and how to use them to process a response.
If you use the response handler, all the HTTP connections will be released automatically.
Creating a response handler
The HttpClient API provides an interface known as ResponseHandler in the package org.apache.http.client. In order to create a response handler, implement this interface and override its handleResponse() method.
Every response has a status code and if the status code is in between 200 and 300, that means the action was successfully received, understood, and accepted. Therefore, in our example, we will handle the entities of the responses with such status codes.
Executing the request using response handler
Follow the steps given below to execute the request using a response handler.
Step 1 - Create an HttpClient Object
The createDefault() method of the HttpClients class returns an object of the class CloseableHttpClient, which is the base implementation of the HttpClient interface. Using this method create an HttpClient object
CloseableHttpClient httpclient = HttpClients.createDefault();
Step 2 - Instantiate the Response Handler
Instantiate the response handler object created above using the following line of code −
ResponseHandler<String> responseHandler = new MyResponseHandler();
Step 3 - Create a HttpGet Object
The HttpGet class represents the HTTP GET request which retrieves the information of the given server using a URI.
Create an HttpGet request by instantiating the HttpGet class and by passing a string representing the URI as a parameter to its constructor.
ResponseHandler<String> responseHandler = new MyResponseHandler();
Step 4 - Execute the Get request using response handler
The CloseableHttpClient class has a variant of execute() method which accepts two objects ResponseHandler and HttpUriRequest, and returns a response object.
String httpResponse = httpclient.execute(httpget, responseHandler);
Example
Following example demonstrates the usage of response handlers.
import java.io.IOException; import org.apache.http.HttpEntity; import org.apache.http.HttpResponse; import org.apache.http.client.ResponseHandler; import org.apache.http.client.methods.HttpGet; import org.apache.http.impl.client.CloseableHttpClient; import org.apache.http.impl.client.HttpClients; import org.apache.http.util.EntityUtils; class MyResponseHandler implements ResponseHandler<String>{ public String handleResponse(final HttpResponse response) throws IOException{ //Get the status of the response int status = response.getStatusLine().getStatusCode(); if (status >= 200 && status < 300) { HttpEntity entity = response.getEntity(); if(entity == null) { return ""; } else { return EntityUtils.toString(entity); } } else { return ""+status; } } } public class ResponseHandlerExample { public static void main(String args[]) throws Exception{ //Create an HttpClient object CloseableHttpClient httpclient = HttpClients.createDefault(); //instantiate the response handler ResponseHandler<String> responseHandler = new MyResponseHandler(); //Create an HttpGet object HttpGet httpget = new HttpGet("https://www.tutorialspoint.com/"); //Execute the Get request by passing the response handler object and HttpGet object String httpresponse = httpclient.execute(httpget, responseHandler); System.out.println(httpresponse); } }
Output
The above programs generate the following output −
<!DOCTYPE html> <!--[if IE 8]><html class = "ie ie8"> <![endif]--> <!--[if IE 9]><html class = "ie ie9"> <![endif]--> <!--[if gt IE 9]><!--> <html lang = "en-US"> <!--<![endif]--> <head> <!-- Basic --> <meta charset = "utf-8"> <meta http-equiv = "X-UA-Compatible" content = "IE = edge"> <meta name = "viewport" content = "width = device-width,initial-scale = 1.0,userscalable = yes"> <link href = "https://cdn.muicss.com/mui-0.9.39/extra/mui-rem.min.css" rel = "stylesheet" type = "text/css" /> <link rel = "stylesheet" href = "/questions/css/home.css?v = 3" /> <script src = "/questions/js/jquery.min.js"></script> <script src = "/questions/js/fontawesome.js"></script> <script src = "https://cdn.muicss.com/mui-0.9.39/js/mui.min.js"></script> </head> . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . <script> window.dataLayer = window.dataLayer || []; function gtag() {dataLayer.push(arguments);} gtag('js', new Date()); gtag('config', 'UA-232293-17'); </script> </body>