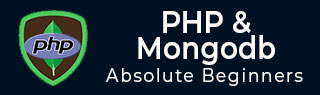
- PHP & MongoDB Tutorial
- PHP & MongoDB - Home
- PHP & MongoDB - Overview
- PHP & MongoDB - Environment Setup
- PHP & MongoDB Examples
- PHP & MongoDB - Connect Database
- PHP & MongoDB - Show Databases
- PHP & MongoDB - Drop Database
- PHP & MongoDB - Create Collection
- PHP & MongoDB - Drop Collection
- PHP & MongoDB - Display Collections
- PHP & MongoDB - Insert Document
- PHP & MongoDB - Select Document
- PHP & MongoDB - Update Document
- PHP & MongoDB - Delete Document
- PHP & MongoDB - Embedded Documents
- PHP & MongoDB - Error Handling
- PHP & MongoDB - Limiting Records
- PHP & MongoDB - Sorting Records
- PHP & MongoDB Useful Resources
- PHP & MongoDB - Quick Guide
- PHP & MongoDB - Useful Resources
- PHP & MongoDB - Discussion
PHP & MongoDB - Quick Guide
PHP & MongoDB - Overview
PHP developer team has provided MongoDB Driver for PHP and have various resources available for it.
First step in connecting to MongoDB using PHP is to have mongodb PHP driver dll in php ext directory and enable it in php.ini and then use mongodb API to connect to the database.
Connecting to MongoDB database
Suppose, MongoDB is installed locally and using default port then following syntax connects to MongoDB database.
$manager = new MongoDB\Driver\Manager("mongodb://localhost:27017");
MongoDB Driver Manager is the central class to do all the operations on mongodb database. To connect to mongodb database, we connect to database by first preparing a command and then executing it.
$statistics = new MongoDB\Driver\Command(["dbstats" => 1]); $cursor = $manager->executeCommand("mydb", $statistics);
Once command is executed, if mydb database is not present, it will be created otherwise, it will be connected.
PHP & MongoDB - Environment Setup
Install MongoDB database
Follow the MongoDB installation steps using MongoDB - Environment Setup
Install PHP
Follow the PHP installation steps using PHP 7 - Environment Setup
PHP MongoDB Driver
To use MongoDB with PHP, you need to use MongoDB PHP driver. Download the driver from the url Download PHP Driver. Make sure to download the latest release of it. Now unzip the archive and put php_mongo.dll in your PHP extension directory ("ext" by default) and add the following line to your php.ini file −
extension = php_mongo.dll
In case of Windows, make sure libsasl.dll is in the windows's PATH. This dll is available in PHP installation directory.
PHP & MongoDB - Connecting Database
First step to do any operation is to create a Manager instance.
// Connect to MongoDB using Manager Instance $manager = new MongoDB\Driver\Manager("mongodb://localhost:27017");
Second step is to prepare and execute a command on a database if the database doesn't exist then MongoDB creates it automatically.
// Create a Command Instance $statistics = new MongoDB\Driver\Command(["dbstats" => 1]); // Execute the command on the database $cursor = $manager->executeCommand("myDb", $statistics);
Example
Try the following example to connect to a MongoDB server −
Copy and paste the following example as mongodb_example.php −
<?php try { // connect to mongodb $manager = new MongoDB\Driver\Manager("mongodb://localhost:27017"); echo "Connection to database successfully"; $statistics = new MongoDB\Driver\Command(["dbstats" => 1]); $cursor = $manager->executeCommand("myDb", $statistics); $statistics = current($cursor->toArray()); echo "<pre>"; print_r($statistics); echo "</pre>"; } catch (MongoDB\Driver\Exception\Exception $e) { echo "Exception:", $e->getMessage(), "\n"; } ?>
Output
Access the mongodb_example.php deployed on apache web server and verify the output.
Connection to database successfully stdClass Object ( [db] => myDb [collections] => 0 [views] => 0 [objects] => 0 [avgObjSize] => 0 [dataSize] => 0 [storageSize] => 0 [totalSize] => 0 [indexes] => 0 [indexSize] => 0 [scaleFactor] => 1 [fileSize] => 0 [fsUsedSize] => 0 [fsTotalSize] => 0 [ok] => 1 )
PHP & MongoDB - Show Databases
First step to do any operation is to create a Manager instance.
// Connect to MongoDB using Manager Instance $manager = new MongoDB\Driver\Manager("mongodb://localhost:27017");
Second step is to prepare and execute a command on a database to show the list of databases available.
// Create a Command Instance $databaseList = new MongoDB\Driver\Command(["listDatabases" => 1]); // Execute the command on the database $cursor = $manager->executeCommand("admin", $databaseList);
Example
Try the following example to list databases available by default in MongoDB server −
Copy and paste the following example as mongodb_example.php −
<?php try { // connect to mongodb $manager = new MongoDB\Driver\Manager("mongodb://localhost:27017"); // Create a Command Instance $databaseList = new MongoDB\Driver\Command(["listDatabases" => 1]); // Execute the command on the database $cursor = $manager->executeCommand("admin", $databaseList); $databases = current($cursor->toArray()); foreach ($databases->databases as $database) { echo $database->name . "<br/>"; } } catch (MongoDB\Driver\Exception\Exception $e) { echo "Exception:", $e->getMessage(), "\n"; } ?>
Output
Access the mongodb_example.php deployed on apache web server and verify the output.
admin config local
PHP & MongoDB - Drop Database
First step to do any operation is to create a Manager instance.
// Connect to MongoDB using Manager Instance $manager = new MongoDB\Driver\Manager("mongodb://localhost:27017");
Second step is to prepare and execute a command to drop the database.
// Create a Command Instance $dropDatabase = new MongoDB\Driver\Command(["dropDatabase" => 1]); // Execute the command on the database $cursor = $manager->executeCommand("myDb", $dropDatabase);
Example
Try the following example to delete a database in MongoDB server −
Copy and paste the following example as mongodb_example.php −
<?php try { // connect to mongodb $manager = new MongoDB\Driver\Manager("mongodb://localhost:27017"); // Create a Command Instance $dropDatabase = new MongoDB\Driver\Command(["dropDatabase" => 1]); // Execute the command on the database $cursor = $manager->executeCommand("myDb", $dropDatabase); echo "Database dropped." } catch (MongoDB\Driver\Exception\Exception $e) { echo "Exception:", $e->getMessage(), "\n"; } ?>
Output
Access the mongodb_example.php deployed on apache web server and verify the output.
Database dropped.
PHP & MongoDB - Create Collection
First step to do any operation is to create a Manager instance.
// Connect to MongoDB using Manager Instance $manager = new MongoDB\Driver\Manager("mongodb://localhost:27017");
Second step is to prepare and execute a command to create the collection.
// Create a Command Instance $createCollection = new MongoDB\Driver\Command(["create" => "sampleCollection"]); // Execute the command on the database $cursor = $manager->executeCommand("myDb", $createCollection);
Example
Try the following example to create a collection in MongoDB server −
Copy and paste the following example as mongodb_example.php −
<?php try { // connect to mongodb $manager = new MongoDB\Driver\Manager("mongodb://localhost:27017"); // Create a Command Instance $createCollection = new MongoDB\Driver\Command(["create" => "sampleCollection"]); // Execute the command on the database $cursor = $manager->executeCommand("myDb", $createCollection); echo "Collection created."; } catch (MongoDB\Driver\Exception\Exception $e) { echo "Exception:", $e->getMessage(), "\n"; } ?>
Output
Access the mongodb_example.php deployed on apache web server and verify the output.
Collection created.
PHP & MongoDB - Drop Collection
First step to do any operation is to create a Manager instance.
// Connect to MongoDB using Manager Instance $manager = new MongoDB\Driver\Manager("mongodb://localhost:27017");
Second step is to prepare and execute a command to drop the collection.
// Create a Command Instance $createCollection = new MongoDB\Driver\Command(["drop" => "sampleCollection"]); // Execute the command on the database $cursor = $manager->executeCommand("myDb", $createCollection);
Example
Try the following example to drop a collection in MongoDB server −
Copy and paste the following example as mongodb_example.php −
<?php try { // connect to mongodb $manager = new MongoDB\Driver\Manager("mongodb://localhost:27017"); // Create a Command Instance $dropCollection = new MongoDB\Driver\Command(["drop" => "sampleCollection"]); // Execute the command on the database $cursor = $manager->executeCommand("myDb", $dropCollection); echo "Collection dropped." } catch (MongoDB\Driver\Exception\Exception $e) { echo "Exception:", $e->getMessage(), "\n"; } ?>
Output
Access the mongodb_example.php deployed on apache web server and verify the output.
Collection dropped.
PHP & MongoDB - Display Collections
First step to do any operation is to create a Manager instance.
// Connect to MongoDB using Manager Instance $manager = new MongoDB\Driver\Manager("mongodb://localhost:27017");
Second step is to prepare and execute a command on a database to show the list of collections available.
// Create a Command Instance $collectionList = new MongoDB\Driver\Command(["listCollections" => 1]); // Execute the command on the database $cursor = $manager->executeCommand("myDb", $collectionList);
Example
Try the following example to list collections of a database in MongoDB server −
Copy and paste the following example as mongodb_example.php −
<?php try { // connect to mongodb $manager = new MongoDB\Driver\Manager("mongodb://localhost:27017"); // Create a Command Instance $collectionList = new MongoDB\Driver\Command(["listCollections" => 1]); // Execute the command on the database $cursor = $manager->executeCommand("myDb", $collectionList); $collections = $cursor->toArray(); foreach ($collections as $collection) { echo $collection->name . "<br/>"; } } catch (MongoDB\Driver\Exception\Exception $e) { echo "Exception:", $e->getMessage(), "\n"; } ?>
Output
Access the mongodb_example.php deployed on apache web server and verify the output.
sampleCollection
PHP & MongoDB - Insert Document
First step to do any operation is to create a Manager instance.
// Connect to MongoDB using Manager Instance $manager = new MongoDB\Driver\Manager("mongodb://localhost:27017");
Second step is to prepare and execute a bulkWrite object to insert record(s) in the collection.
// Create a BulkWrite Object $bulk = new MongoDB\Driver\BulkWrite(['ordered' => true]); $bulk->insert(['First_Name' => "Mahesh", 'Last_Name' => 'Parashar', 'Date_Of_Birth' => '1990-08-21', 'e_mail' => 'mahesh_parashar.123@gmail.com', 'phone' => '9034343345']); // Execute the commands. $result = $manager->executeBulkWrite('myDb.sampleCollection', $bulk);
Example
Try the following example to insert documents in a collection in MongoDB server −
Copy and paste the following example as mongodb_example.php −
<?php try { $bulk = new MongoDB\Driver\BulkWrite(['ordered' => true]); $bulk->insert(['First_Name' => "Mahesh", 'Last_Name' => 'Parashar', 'Date_Of_Birth' => '1990-08-21', 'e_mail' => 'mahesh_parashar.123@gmail.com', 'phone' => '9034343345']); $bulk->insert(['First_Name' => "Radhika", 'Last_Name' => 'Sharma', 'Date_Of_Birth' => '1995-09-26', 'e_mail' => 'radhika_sharma.123@gmail.com', 'phone' => '9000012345']); $bulk->insert(['First_Name' => "Rachel", 'Last_Name' => 'Christopher', 'Date_Of_Birth' => '1990-02-16', 'e_mail' => 'rachel_christopher.123@gmail.com', 'phone' => '9000054321']); $bulk->insert(['First_Name' => "Fathima", 'Last_Name' => 'Sheik', 'Date_Of_Birth' => '1990-02-16', 'e_mail' => 'fathima_sheik.123@gmail.com', 'phone' => '9000012345']); // connect to mongodb $manager = new MongoDB\Driver\Manager("mongodb://localhost:27017"); $result = $manager->executeBulkWrite('myDb.sampleCollection', $bulk); printf("Inserted %d document(s).\n", $result->getInsertedCount()); } catch (MongoDB\Driver\Exception\Exception $e) { echo "Exception:", $e->getMessage(), "\n"; } ?>
Output
Access the mongodb_example.php deployed on apache web server and verify the output.
Inserted 4 document(s).
PHP & MongoDB - Select Document
First step to do any operation is to create a Manager instance.
// Connect to MongoDB using Manager Instance $manager = new MongoDB\Driver\Manager("mongodb://localhost:27017");
Second step is to prepare and execute a Query object to select record(s) in the collection.
// Create a Query Object $query = new MongoDB\Driver\Query(['First_Name' => "Radhika"]); // Execute the query $rows = $manager->executeQuery("testdb.sampleCollection", $query);
Example
Try the following example to select a document in MongoDB server −
Copy and paste the following example as mongodb_example.php −
<?php try { // connect to mongodb $manager = new MongoDB\Driver\Manager("mongodb://localhost:27017"); $filter = []; // Create a Query Object $query = new MongoDB\Driver\Query($filter); // Execute the query $rows = $manager->executeQuery("myDb.sampleCollection", $query); foreach ($rows as $row) { printf("First Name: %s, Last Name: %s.<br/>", $row->First_Name, $row->Last_Name); } } catch (MongoDB\Driver\Exception\Exception $e) { echo "Exception:", $e->getMessage(), "\n"; } ?>
Output
Access the mongodb_example.php deployed on apache web server and verify the output.
First Name: Radhika, Last Name: Sharma. First Name: Rachel, Last Name: Christopher. First Name: Fathima, Last Name: Sheik.
PHP & MongoDB - Update Document
First step to do any operation is to create a Manager instance.
// Connect to MongoDB using Manager Instance $manager = new MongoDB\Driver\Manager("mongodb://localhost:27017");
Second step is to prepare and execute a bulkWrite object to update record(s) in the collection.
// Create a BulkWrite Object $bulk = new MongoDB\Driver\BulkWrite(['ordered' => true]); $bulk->update(['First_Name' => "Mahesh"],['$set' => ['e_mail' => 'maheshparashar@gmail.com']]); // Execute the commands. $result = $manager->executeBulkWrite('myDb.sampleCollection', $bulk);
Example
Try the following example to update a document in MongoDB server −
Copy and paste the following example as mongodb_example.php −
<?php try { $bulk = new MongoDB\Driver\BulkWrite(['ordered' => true]); $bulk->update(['First_Name' => "Mahesh"],['$set' => ['e_mail' => 'maheshparashar@gmail.com']]); // connect to mongodb $manager = new MongoDB\Driver\Manager("mongodb://localhost:27017"); $result = $manager->executeBulkWrite('myDb.sampleCollection', $bulk); printf("Updated %d document(s).\n", $result->getModifiedCount()); } catch (MongoDB\Driver\Exception\Exception $e) { echo "Exception:", $e->getMessage(), "\n"; } ?>
Output
Access the mongodb_example.php deployed on apache web server and verify the output.
Updated 1 document(s).
PHP & MongoDB - Delete Document
First step to do any operation is to create a Manager instance.
// Connect to MongoDB using Manager Instance $manager = new MongoDB\Driver\Manager("mongodb://localhost:27017");
Second step is to prepare and execute a bulkWrite object to delete record(s) in the collection.
// Create a BulkWrite Object $bulk = new MongoDB\Driver\BulkWrite(['ordered' => true]); $bulk->delete(['First_Name' => "Mahesh"]); // Execute the commands. $result = $manager->executeBulkWrite('myDb.sampleCollection', $bulk);
Example
Try the following example to delete a document of a collection in MongoDB server −
Copy and paste the following example as mongodb_example.php −
<?php try { $bulk = new MongoDB\Driver\BulkWrite(['ordered' => true]); $bulk->delete(['First_Name' => "Mahesh"]); // connect to mongodb $manager = new MongoDB\Driver\Manager("mongodb://localhost:27017"); $result = $manager->executeBulkWrite('myDb.sampleCollection', $bulk); echo "Document deleted." } catch (MongoDB\Driver\Exception\Exception $e) { echo "Exception:", $e->getMessage(), "\n"; } ?>
Output
Access the mongodb_example.php deployed on apache web server and verify the output.
Document deleted.
PHP & MongoDB - Insert Embedded Documents
First step to do any operation is to create a Manager instance.
// Connect to MongoDB using Manager Instance $manager = new MongoDB\Driver\Manager("mongodb://localhost:27017");
Second step is to prepare and execute a bulkWrite object to insert record(s) with embedded documents in the collection.
// Create a BulkWrite Object $bulk = new MongoDB\Driver\BulkWrite(['ordered' => true]); $bulk->insert(['title' => "MongoDB Overview", 'description' => 'MongoDB is no SQL database', 'by' => 'tutorials point', 'url' => 'http://www.tutorialspoint.com', 'comments' => [ ['user' => "user1", 'message' => "My First Comment", 'dateCreated' => "20/2/2020", 'like' => 0], ['user' => "user2", 'message' => "My Second Comment", 'dateCreated' => "20/2/2020", 'like' => 0], ]]); // Execute the commands. $result = $manager->executeBulkWrite('myDb.sampleCollection', $bulk);
Example
Try the following example to insert document with embedded documents in a collection in MongoDB server −
Copy and paste the following example as mongodb_example.php −
<?php try { // connect to mongodb $manager = new MongoDB\Driver\Manager("mongodb://localhost:27017"); $bulk = new MongoDB\Driver\BulkWrite(['ordered' => true]); $bulk->insert(['title' => "MongoDB Overview", 'description' => 'MongoDB is no SQL database', 'by' => 'tutorials point', 'url' => 'http://www.tutorialspoint.com', 'comments' => [ ['user' => "user1", 'message' => "My First Comment", 'dateCreated' => "20/2/2020", 'like' => 0], ['user' => "user2", 'message' => "My Second Comment", 'dateCreated' => "20/2/2020", 'like' => 0], ]]); $result = $manager->executeBulkWrite('myDb.sampleCollection', $bulk); printf("Inserted %d document(s).\n", $result->getInsertedCount()); } catch (MongoDB\Driver\Exception\Exception $e) { echo "Exception:", $e->getMessage(), "\n"; } ?>
Output
Access the mongodb_example.php deployed on apache web server and verify the output.
Inserted 1 document(s).
PHP & MongoDB - Error Handling
MongoDB driver operations throws exceptions in case of any database operations and can be captured easily using following syntax:
try { } catch (MongoDB\Driver\Exception\Exception $e) { echo "Exception:", $e->getMessage(), "<br/>"; echo "File:", $e->getFile(), "<br/>"; echo "Line:", $e->getLine(), "<br/>"; }
Example
Try the following example to check database operation error in MongoDB server −
Copy and paste the following example as mongodb_example.php −
<?php try { // connect to mongodb $manager = new MongoDB\Driver\Manager("mongodb://localhost:27017"); // Create a Command Instance $createCollection = new MongoDB\Driver\Command(["create" => "sampleCollection"]); // Execute the command on the database $cursor = $manager->executeCommand("myDb", $createCollection); echo "Collection created."; } catch (MongoDB\Driver\Exception\Exception $e) { echo "Exception:", $e->getMessage(), "<br/>"; echo "File:", $e->getFile(), "<br/>"; echo "Line:", $e->getLine(), "<br/>"; } ?>
Output
Access the mongodb_example.php deployed on apache web server and verify the output.
Exception:Collection already exists. NS: myDb.sampleCollection File: \htdocs\php_mongodb\mongodb_example.php Line:10
PHP & MongoDB - Limit Records
First step to do any operation is to create a Manager instance.
// Connect to MongoDB using Manager Instance $manager = new MongoDB\Driver\Manager("mongodb://localhost:27017");
Second step is to prepare and execute a Query object to record(s) in the collection and pass it filters and options to limit search results.
$filter = []; $options = ['limit' => 1]; // Create a Query Object $query = new MongoDB\Driver\Query($filter, $options); // Execute the query $rows = $manager->executeQuery("testdb.sampleCollection", $query);
Example
Try the following example to limit search results in MongoDB server −
Copy and paste the following example as mongodb_example.php −
<?php try { // connect to mongodb $manager = new MongoDB\Driver\Manager("mongodb://localhost:27017"); $filter = []; $options = ['limit' => 1]; // Create a Query Object $query = new MongoDB\Driver\Query($filter, $options); // Execute the query $rows = $manager->executeQuery("myDb.sampleCollection", $query); foreach ($rows as $row) { printf("First Name: %s, Last Name: %s.<br/>", $row->First_Name, $row->Last_Name); } } catch (MongoDB\Driver\Exception\Exception $e) { echo "Exception:", $e->getMessage(), "\n"; } ?>
Output
Access the mongodb_example.php deployed on apache web server and verify the output.
First Name: Radhika, Last Name: Sharma.
PHP & MongoDB - Sorting Records
First step to do any operation is to create a Manager instance.
// Connect to MongoDB using Manager Instance $manager = new MongoDB\Driver\Manager("mongodb://localhost:27017");
Second step is to prepare and execute a Query object to select record(s) in the collection and pass it filters and options to sort the records.
$filter = []; // Sort in Descending Order, For ascending order pass 1 $options = ['sort' => ['First_Name' => -1]]; // Create a Query Object $query = new MongoDB\Driver\Query($filter, $options); // Execute the query $rows = $manager->executeQuery("testdb.sampleCollection", $query);
Example
Try the following example to limit search results in MongoDB server −
Copy and paste the following example as mongodb_example.php −
<?php try { // connect to mongodb $manager = new MongoDB\Driver\Manager("mongodb://localhost:27017"); $filter = []; $options = ['limit' => 3, 'sort' => ['First_Name' => -1]]; // Create a Query Object $query = new MongoDB\Driver\Query($filter, $options); // Execute the query $rows = $manager->executeQuery("myDb.sampleCollection", $query); foreach ($rows as $row) { printf("First Name: %s, Last Name: %s.<br/>", $row->First_Name, $row->Last_Name); } } catch (MongoDB\Driver\Exception\Exception $e) { echo "Exception:", $e->getMessage(), "\n"; } ?>
Output
Access the mongodb_example.php deployed on apache web server and verify the output.
First Name: Radhika, Last Name: Sharma. First Name: Rachel, Last Name: Christopher. First Name: Fathima, Last Name: Sheik.
To Continue Learning Please Login