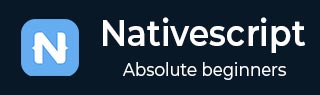
- NativeScript Tutorial
- NativeScript - Home
- NativeScript - Introduction
- NativeScript - Installation
- NativeScript - Architecture
- NativeScript - Angular Application
- NativeScript - Templates
- NativeScript - Widgets
- NativeScript - Layout Containers
- NativeScript - Navigation
- NativeScript - Events Handling
- NativeScript - Data Binding
- NativeScript - Modules
- NativeScript - Plugins
- NativeScript - Native APIs Using JavaScript
- NativeScript - Creating an Application in Android
- NativeScript - Creating an Application in iOS
- NativeScript - Testing
- NativeScript Useful Resources
- NativeScript - Quick Guide
- NativeScript - Useful Resources
- NativeScript - Discussion
NativeScript - Architecture
NativeScript is an advanced framework to create mobile application. It hides the complexity of creating mobile application and exposes a rather simple API to create highly optimized and advanced mobile application. NativeScript enables even entry level developers to easily create mobile application in both Android and iOS.
Let us understand the architecture of the NativeScript framework in this chapter.
Introduction
The core concept of NativeScript framework is to enable the developer to create hybrid style mobile application. Hybrid application uses the platform specific browser API to host a web application inside a normal mobile application and provides system access to the application through JavaScript API.
NativeScript invests heavily on the JavaScript language to provide an efficient framework for developers. Since JavaScript is de-facto standard for client side programming (Web development) and every developer is well aware of the JavaScript language, it helps developers to easily get into the NativeScript framework. At the low level, NativeScript exposes the native API through a collection of JavaScript plugins called Native plugins.
NativeScript builds on the foundation of Native plugins and provides many high level and easy to use JavaScript Modules. Each module does a specific functionality like accessing a camera, designing a screen, etc. All these modules can be combined in multiple ways to architect a complex mobile application.
Below diagram shows the high level overview of the NativeScript framework −

NativeScript Application − NativeScript framework allows developer to use either Angular style application or Vue Style application.
JavaScript Modules − NativeScript framework provides a rich set of JavaScript modules clearly categorized as UI Modules, Application Modules, Core Modules, etc. All modules can be accessed by application at any time to write any level of complex application.
JavaScript plugins − NativeScript framework provides a large collection of JavaScript plugins to access the platform related functionality. Modules uses the JavaScript plugins to provide platform specific functionality.
Native plugins− Native plugins are written in platform specific language to wrapper the system functionality which will be further used by JavaScript plugin.
Platform API − API provided by platform vendors.
In short, NativeScript application is written and organized using modules. Modules are written in pure JavaScript and the modules access the platform related functionality (whenever needed) through plugins and finally, the plugins bridge the platform API and JavaScript API.
Workflow of a NativeScript Application
As we learned earlier, NativeScript application is composed of modules. Each and every module enables a specific feature. The two important categories of module to bootstrap a NativeScript application are as follows −
Root Modules
Page Modules
Root and Page modules can be categorized as application modules. The application module is the entry point of the NativeScript application. It bootstraps a page, enables the developer to create user interface of the page and finally allows execution of the business logic of the page. An application module consists of below three items −
User interface design coded in XML (e.g. page.xml/page.component.html)
Styles coded in CSS (e.g. page.css/page.component.css)
Actual business logic of the module in JavaScript (e.g. page.js/page.component.ts)
NativeScript provides lot of UI components (under UI Module) to design the application page. UI Component can be represented in XML format or HTML format in Angular based application. Application module uses the UI Component to design the page and store the design in separate XML, page.xml/page.component.html. The design can be styled using standard CSS.
Application modules stores the style of the design in separate CSS, page.css/page.component.css. The functionality of the page can be done using JavaScript/TypeScript, which has full access to the design as well as the platform features. Application module uses a separate file, page.js/page.component.ts to code the actual functionality of the page.
Root Modules
NativeScript manages the user interface and user interaction through UI containers. Every UI container should have a Root Module and through which the UI container manages UI. NativeScript application have two type of UI containers −
Application Container − Every NativeScript application should have one application container and it will be set using application.run() method. It initializes the UI of the application.
Model View Container − NativeScript manages the Modal dialogs using model view container. A NativeScript application can have any number of model view container.
Every root module should have only one UI Component as its content. The UI component in turn can have other UI components as its children. NativeScript provides a lot of UI component like TabView, ScrollView, etc., with child feature. We can use these as root UI component. One exception is Frame, which does not have child option but can be used as root component. Frame provides options to load Page Modules and options to navigate to other page modules as well.
Page Modules
In NativeScript, each and every page is basically a Page Module. Page module is designed using the rich set of UI components provided by NativeScript. Page modules are loadedinto the application through Frame component (using its defaultPage attribute or using navigate() method), which in turn loaded using Root Modules, which again in turn loaded using application.run() while the application is started.
The work flow of the application can be represented as in the below diagram −

The above diagram is explained in detail in the following steps −
NativeScript Application starts and calls application.run() method.
application.run() loads a Root module.
Root Module is designed using any one of the UI component as specified below −
Frame
TabView
SideDrawer
Any Layout View
Frame component loads the specified page (Page module) and gets rendered. Other UI components will be rendered as specified in the Root Module. Other UI component also has option to load Page Modules as its main content.
Workflow of Angular based NativeScript Application
As we learned earlier, NativeScript framework provides multiple methodologies to cater different category of developers. The methodologies supported by NativeScript are as follows −
NativeScript Core − Basic or core concept of NativeScript Framework
Angular + NativeScript − Angular based methodology
Vuejs + NativeScript − Vue.js based methodology
Let us learn how Angular framework is incorporated into the NativeScript framework.
Step 1
NativeScript provides an object (platformNativeScriptDynamic) to bootstrap the Angular application. platformNativeScriptDynamic has a method, bootstrapModule, which is used to start the application.
The syntax to bootstrap the application using Angular framework is as follows −
import { platformNativeScriptDynamic } from "nativescript-angular/platform"; import { AppModule } from "./app/app.module"; platformNativeScriptDynamic().bootstrapModule(AppModule);
Here,
AppModule is our Root module.
Step 2
A simple implementation (below specified code) of the app module.
import { NgModule } from "@angular/core"; import { NativeScriptModule } from "nativescript-angular/nativescript.module"; import { AppRoutingModule } from "./app-routing.module"; import { AppComponent } from "./app.component"; @NgModule( { bootstrap: [ AppComponent ], imports: [ NativeScriptModule, AppRoutingModule ], declarations: [ AppComponent ] } ) export class AppModule { }
Here,
AppModule starts the application by loading AppComponent component. Angular components are similar to pages and are used for both design and programming logic.
A simple implementation of AppComponent (app.component.ts) and its presentation logic (app.component.css) is as follows −
app.component.ts
import { Component } from "@angular/core"; @Component( { selector: "ns-app", templateUrl: "app.component.html" } ) export class AppComponent { }
Here,
templateUrl refers the design of the component.
app.component.html
<page-router-outlet></page-router-outlet>
Here,
page-router-outlet is the place where the Angular application get attached.
In summary, Angular framework is composed of modules similar to NativeScript framework with slight differences. Each module in the Angular will have an Angular component and a router setup file (page-routing.mocdule.ts). The router is set per module and it takes care of navigation. Angular components are analogues to pages in NativeSctipt core.
Each component will have a UI design (page.component.html), a style sheet (page.component.css), and a JavaScript/TypeScript code file (page.component.ts).
To Continue Learning Please Login