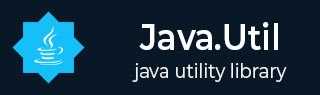
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java UUID toString() Method
Description
The Java UUID toString() method is used to return a String object representing this UUID.
Declaration
Following is the declaration for java.util.UUID.toString() method.
public String toString()
Parameters
NA
Return Value
The method call returns a string representation of this UUID.
Exception
NA
Getting String Representation of a UUID generated using Standard Formatted String Example
The following example shows the usage of Java UUID toString() method to get the string representation of this UUID. We've created a UUID object using a given string. Then we've printed the string representation of this UUID object using toString() method.
package com.tutorialspoint; import java.util.UUID; public class UUIDDemo { public static void main(String[] args) { // creating UUID UUID x = UUID.fromString("38400000-8cf0-11bd-b23e-10b96e4ef00d"); // getting string representation System.out.println("string representation: "+x.toString()); } }
Output
Let us compile and run the above program, this will produce the following result.
string representation: 38400000-8cf0-11bd-b23e-10b96e4ef00d
Getting String Representation of a Random UUID generated Example
The following example shows usage of Java UUID toString() method to get the string representation of this UUID. We've created a UUID object using randomUUID() method. Then we've printed the string representation of this UUID object using toString() method.
package com.tutorialspoint; import java.util.UUID; public class UUIDDemo { public static void main(String[] args) { // creating UUID UUID x = UUID.randomUUID(); // getting string representation System.out.println("string representation: "+x.toString()); } }
Output
Let us compile and run the above program, this will produce the following result.
string representation: 8f5f2803-2c9b-4c6c-90d4-6d53828d245b
Getting String Representation of a UUID generated using Bytes Example
The following example shows usage of Java UUID toString() method to get the string representation of this UUID. We've created a UUID object using nameUUIDFromBytes() method. Then we've printed the string representation of this UUID object using toString() method.
package com.tutorialspoint; import java.util.UUID; public class UUIDDemo { public static void main(String[] args) { // creating byte array byte[] nbyte = {10,20,30}; // creating UUID from byte UUID uid = UUID.nameUUIDFromBytes(nbyte); // getting string representation System.out.println("string representation: "+uid.toString()); } }
Output
Let us compile and run the above program, this will produce the following result.
string representation: 7f49b84d-0bbc-38e9-a493-718013baace6
To Continue Learning Please Login