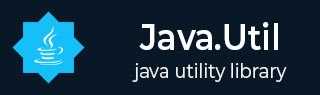
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java PropertyPermission Class
Introduction
The Java PropertyPermission class is a class for property permissions. Following are the important points about PropertyPermission −
The name is the name of the property ("java.home", "os.name", etc).
The naming convention follows the hierarchical property naming convention.An asterisk may appear at the end of the name, following a ".", or by itself, to signify a wildcard match.For example: "java.*" or "*" is valid, "*java" or "a*b" is not valid.
Class declaration
Following is the declaration for java.util.PropertyPermission class −
public final class PropertyPermission extends BasicPermission
Class constructors
Sr.No. | Constructor & Description |
---|---|
1 | PropertyPermission(String name, String actions) This creates a new PropertyPermission object with the specified name. |
Class methods
Sr.No. | Method & Description |
---|---|
1 | boolean equals(Object obj)
This method checks two PropertyPermission objects for equality. |
2 | String getActions()
This method returns the "canonical string representation" of the actions. |
3 | int hashCode()
This method returns the hash code value for this object. |
4 | boolean implies(Permission p)
This method checks if this PropertyPermission object "implies" the specified permission. |
5 | PermissionCollection newPermissionCollection()
This method returns a returns a new PermissionCollection object for storing PropertyPermission objects. |
Methods inherited
This class inherits methods from the following classes −
- java.util.Permission
- java.util.Object
Checking a PropertyPermission Object for read/write actions Example
The following example shows the usage of Java PropertyPermission getActions() method to check a permission object. We've built a PropertyPermission object, and then check the permission to be read or write.
package com.tutorialspoint; import java.util.PropertyPermission; public class PropertyPermissionDemo { private static PropertyPermission permission; public static void main(String[] args) { // Build property permissions permission = new PropertyPermission("java.home.*", "read,write"); // Check permissions checkFilePermissions("java.home.usr"); } private static void checkFilePermissions(String path) { // Check permission given name if(path.matches(permission.getName())) { // Get actions list String actions = permission.getActions(); // Match read write actions if(actions.contains("read")) System.out.println("Has permissions on "+path+" for read"); if(actions.contains("write")) System.out.println("Has permissions on "+path+" for write"); } } }
Output
Let us compile and run the above program, this will produce the following result −
Has permissions on java.home.usr for read Has permissions on java.home.usr for write
To Continue Learning Please Login