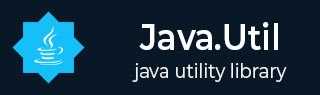
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java Scanner match() Method
Description
The java Scanner match() method returns the match result of the last scanning operation performed by this scanner. This method throws IllegalStateException if no match has been performed, or if the last match was not successful.
Declaration
Following is the declaration for java.util.Scanner.match() method
public MatchResult match()
Parameters
NA
Return Value
This method returns a match result for the last match operation
Exception
IllegalStateException − If no match result is available
Getting Last Match Result of a Scanner on a String Example
The following example shows the usage of Java Scanner match() method to get the match result for last match operation. We've created a scanner object using a given string. We've checked a string pattern "Hello" to be present in the strin using hasNext() method and using match(), the result is printed. Then we printed the string using nextLine() method and then scanner is closed using close() method.
package com.tutorialspoint; import java.util.Scanner; public class ScannerDemo { public static void main(String[] args) { String s = "Hello World! 3 + 3.0 = 6 "; // create a new scanner with the specified String Object Scanner scanner = new Scanner(s); // check if next token is "Hello" System.out.println(scanner.hasNext("Hello")); // find the last match and print it System.out.println(scanner.match().group()); // print the line System.out.println(scanner.nextLine()); // close the scanner scanner.close(); } }
Output
Let us compile and run the above program, this will produce the following result −
true Hello Hello World! 3 + 3.0 = 6
Getting Last Match Result of a Scanner on User Input Example
The following example shows the usage of Java Scanner match() method to get the match result for last match operation. We've created a scanner object using System.in class. We've checked a string pattern "Hello" to be present in the strin using hasNext() method and using match(), the result is printed. Then we printed the string using nextLine() method and then scanner is closed using close() method.
package com.tutorialspoint; import java.util.Scanner; public class ScannerDemo { public static void main(String[] args) { // create a new scanner with the System Input Scanner scanner = new Scanner(System.in); // check if next token is "Hello" System.out.println(scanner.hasNext("Hello")); // find the last match and print it System.out.println(scanner.match().group()); // print the line System.out.println(scanner.nextLine()); // close the scanner scanner.close(); } }
Output
Let us compile and run the above program, this will produce the following result −
Hello World true Hello Hello World
Getting Last Match Result of a Scanner on a Properties File Example
The following example shows the usage of Java Scanner match() method to get the match result for last match operation. We've created a scanner object using a File properties.txt. We've checked a string pattern "Hello" to be present in the strin using hasNext() method and using match(), the result is printed. Then we printed the string using nextLine() method and then scanner is closed using close() method.
package com.tutorialspoint; import java.io.File; import java.io.FileNotFoundException; import java.util.Scanner; public class ScannerDemo { public static void main(String[] args) throws FileNotFoundException { // create a new scanner with a file as input Scanner scanner = new Scanner(new File("properties.txt")); // check if next token is "Hello" System.out.println(scanner.hasNext("Hello")); // find the last match and print it System.out.println(scanner.match().group()); // print the line System.out.println(scanner.nextLine()); // close the scanner scanner.close(); } }
Assuming we have a file properties.txt available in your CLASSPATH, with the following content. This file will be used as an input for our example program −
Hello World! 3 + 3.0 = 6
Output
Let us compile and run the above program, this will produce the following result −
true Hello Hello World! 3 + 3.0 = 6
To Continue Learning Please Login