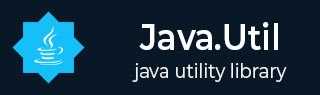
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
java Random longs() Method
Description
The java Random longs() method returns an effectively unlimited stream of pseudorandom long values. A pseudorandom long value is generated as if it's the result of calling the method nextLong().
Declaration
Following is the declaration for java.util.Random.longs() method.
public LongStream longs()
Parameters
NA
Return Value
The method call returns a stream of pseudorandom long values.
Exception
NA
java Random longs(long randomNumberOrigin, long randomNumberBound) Method
Description
The java Random longs(long randomNumberOrigin, long randomNumberBound) method returns an effectively unlimited stream of pseudorandom long values, each conforming to the given origin (inclusive) and bound (exclusive).
Declaration
Following is the declaration for java.util.Random.longs(long randomNumberOrigin, long randomNumberBound) method.
public LongStream longs(long randomNumberOrigin, long randomNumberBound)
Parameters
randomNumberOrigin − the origin (inclusive) of each random value
randomNumberBound − the bound (exclusive) of each random value
Return Value
The method call a stream of pseudorandom long values, each with the given origin (inclusive) and bound (exclusive).
Exception
IllegalArgumentException − if randomNumberOrigin is greater than or equal to randomNumberBound
java Random longs(long streamSize) Method
Description
The java Random longs(long streamSize) method returns a stream producing the given streamSize number of pseudorandom long values. A pseudorandom long value is generated as if it's the result of calling the method nextLong().
Declaration
Following is the declaration for java.util.Random.longs(long streamSize) method.
public LongStream longs(long streamSize)
Parameters
long streamSize − the number of values to generate.
Return Value
The method call a stream of long values.
Exception
IllegalArgumentException − if streamSize is less than zero.
java Random longs(long streamSize, long randomNumberOrigin, long randomNumberBound) Method
Description
The java Random longs(long streamSize, long randomNumberOrigin, long randomNumberBound) method returns a stream producing the given streamSize number of pseudorandom long values, each conforming to the given origin (inclusive) and bound (exclusive).
Declaration
Following is the declaration for java.util.Random.longs(long streamSize, long randomNumberOrigin, long randomNumberBound) method.
public LongStream longs(long streamSize, long randomNumberOrigin, long randomNumberBound)
Parameters
long streamSize − the number of values to generate.
Return Value
The method call a stream of long values.
Exception
IllegalArgumentException − if streamSize is less than zero.
Getting a Stream of Random Long Numbers Example
The following example shows the usage of Java Random longs() method. Firstly, we've created a Random object and then using longs() we retrieved a stream of random long values and printed first value.
package com.tutorialspoint; import java.util.Random; import java.util.stream.LongStream; public class RandomDemo { public static void main( String args[] ) { // create random object LongStream randomNoStream = new Random().longs(); // print a random long value randomNoStream.limit(1).forEach( i -> System.out.println(i)); } }
Output
Let us compile and run the above program, this will produce the following result.
1970507372760910376
Getting a Stream of Random Long Numbers Between Given Two Numbers Example
The following example shows the usage of Java Random longs(long randomNumberOrigin, long randomNumberBound) method. Firstly, we've created a Random object and then using longs(long randomNumberOrigin, long randomNumberBound) we retrieved a stream of random long values and printed first value.
package com.tutorialspoint; import java.util.Random; import java.util.stream.LongStream; public class RandomDemo { public static void main( String args[] ) { // create random object LongStream randomNoStream = new Random().longs(5,6); // print a random long value randomNoStream.limit(1).forEach( i -> System.out.println(i)); } }
Output
Let us compile and run the above program, this will produce the following result.
5
Getting a Stream of Random Long Numbers of Given Size Example
The following example shows the usage of Java Random longs(long streamSize) method. Firstly, we've created a Random object and then using longs(long streamSize) we retrieved a stream of a random long value and printed its value.
package com.tutorialspoint; import java.util.Random; import java.util.stream.LongStream; public class RandomDemo { public static void main( String args[] ) { // create random object LongStream randomNoStream = new Random().longs(1); // print a random long value randomNoStream.forEach( i -> System.out.println(i)); } }
Output
Let us compile and run the above program, this will produce the following result.
4483414426301553028
Getting a Stream of Random Long Numbers of Given Size Between Given Two Numbers Example
The following example shows the usage of Java Random longs(long streamSize, long randomNumberOrigin, long randomNumberBound) method. Firstly, we've created a Random object and then using longs(long streamSize, long randomNumberOrigin, long randomNumberBound) we retrieved a stream of a random long value and printed its value.
package com.tutorialspoint; import java.util.Random; import java.util.stream.LongStream; public class RandomDemo { public static void main( String args[] ) { // create random object LongStream randomNoStream = new Random().longs(1,5,6); // print a random long value randomNoStream.forEach( i -> System.out.println(i)); } }
Output
Let us compile and run the above program, this will produce the following result.
5
To Continue Learning Please Login