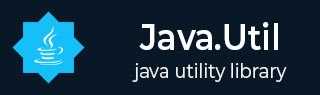
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
java Random ints() Method
Description
The java Random ints() method returns an effectively unlimited stream of pseudorandom int values. A pseudorandom int value is generated as if it's the result of calling the method nextInt().
Declaration
Following is the declaration for java.util.Random.ints() method.
public IntStream ints()
Parameters
NA
Return Value
The method call returns a stream of pseudorandom int values.
Exception
NA
java Random ints(int randomNumberOrigin, int randomNumberBound) Method
Description
The java Random ints(int randomNumberOrigin, int randomNumberBound) method returns an effectively unlimited stream of pseudorandom int values, each conforming to the given origin (inclusive) and bound (exclusive).
Declaration
Following is the declaration for java.util.Random.ints(int randomNumberOrigin, int randomNumberBound) method.
public IntStream ints(int randomNumberOrigin, int randomNumberBound)
Parameters
randomNumberOrigin − the origin (inclusive) of each random value
randomNumberBound − the bound (exclusive) of each random value
Return Value
The method call a stream of pseudorandom int values, each with the given origin (inclusive) and bound (exclusive).
Exception
IllegalArgumentException − if randomNumberOrigin is greater than or equal to randomNumberBound
java Random ints(long streamSize) Method
Description
The java Random ints(long streamSize) method returns a stream producing the given streamSize number of pseudorandom int values. A pseudorandom int value is generated as if it's the result of calling the method nextInt().
Declaration
Following is the declaration for java.util.Random.ints(long streamSize) method.
public IntStream ints(long streamSize)
Parameters
long streamSize − the number of values to generate.
Return Value
The method call a stream of int values.
Exception
IllegalArgumentException − if streamSize is less than zero.
java Random ints(long streamSize, int randomNumberOrigin, int randomNumberBound) Method
Description
The java Random ints(long streamSize, int randomNumberOrigin, int randomNumberBound) method returns a stream producing the given streamSize number of pseudorandom int values, each conforming to the given origin (inclusive) and bound (exclusive).
Declaration
Following is the declaration for java.util.Random.ints(long streamSize, int randomNumberOrigin, int randomNumberBound) method.
public IntStream ints(long streamSize, int randomNumberOrigin, int randomNumberBound)
Parameters
long streamSize − the number of values to generate.
Return Value
The method call a stream of int values.
Exception
IllegalArgumentException − if streamSize is less than zero.
Getting a Stream of Random Integer Numbers Example
The following example shows the usage of Java Random ints() method. Firstly, we've created a Random object and then using ints() we retrieved a stream of random int values and printed first value.
package com.tutorialspoint; import java.util.Random; import java.util.stream.IntStream; public class RandomDemo { public static void main( String args[] ) { // create random object IntStream randomNoStream = new Random().ints(); // print a random int value randomNoStream.limit(1).forEach( i -> System.out.println(i)); } }
Output
Let us compile and run the above program, this will produce the following result.
1809143726
Getting a Stream of Random Integer Numbers Between Given Two Numbers Example
The following example shows the usage of Java Random ints(int randomNumberOrigin, int randomNumberBound) method. Firstly, we've created a Random object and then using ints(int randomNumberOrigin, int randomNumberBound) we retrieved a stream of random int values and printed first value.
package com.tutorialspoint; import java.util.Random; import java.util.stream.IntStream; public class RandomDemo { public static void main( String args[] ) { // create random object IntStream randomNoStream = new Random().ints(5,6); // print a random int value randomNoStream.limit(1).forEach( i -> System.out.println(i)); } }
Output
Let us compile and run the above program, this will produce the following result.
5
Getting a Stream of Random Integer Numbers of Given Size Example
The following example shows the usage of Java Random ints(long streamSize) method. Firstly, we've created a Random object and then using ints(long streamSize) we retrieved a stream of a random int value and printed its value.
package com.tutorialspoint; import java.util.Random; import java.util.stream.IntStream; public class RandomDemo { public static void main( String args[] ) { // create random object IntStream randomNoStream = new Random().ints(1); // print a random int value randomNoStream.forEach( i -> System.out.println(i)); } }
Output
Let us compile and run the above program, this will produce the following result.
1075056095
Getting a Stream of Random Integers Numbers of Given Size Between Given Two Numbers Example
The following example shows the usage of Java Random ints(long streamSize, int randomNumberOrigin, int randomNumberBound) method. Firstly, we've created a Random object and then using ints(long streamSize, int randomNumberOrigin, int randomNumberBound) we retrieved a stream of a random int value and printed its value.
package com.tutorialspoint; import java.util.Random; import java.util.stream.IntStream; public class RandomDemo { public static void main( String args[] ) { // create random object IntStream randomNoStream = new Random().ints(1,5,6); // print a random int value randomNoStream.forEach( i -> System.out.println(i)); } }
Output
Let us compile and run the above program, this will produce the following result.
5
To Continue Learning Please Login