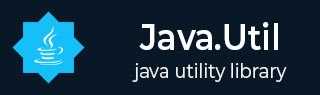
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java Locale lookupTag() Method
Description
The Java Locale lookupTag(List<Locale.LanguageRange> priorityList, Collection<String> tags) method returns the best-matching language tag using the lookup mechanism defined in RFC 4647. This lookup operation on the given tags ensures that the first matching tag with preserved case is returned.
Declaration
Following is the declaration for java.util.Locale.lookupTag() method
public static String lookupTag​(List<Locale.LanguageRange> priorityList, Collection<String> tags)
Parameters
priorityList − user's Language Priority List in which each language tag is sorted in descending order based on priority or weight.
tags − language tags.
Return Value
This method returns the best matching language tag chosen based on priority or weight, or null if nothing matches.
Exception
NullPointerException − if priorityList or locales is null
Getting Best Matched Language Tag for en, jp Locales Example
The following example shows the usage of Java Locale lookupTag() method. We're creating a string of comma separated locales and using that string, we've created a locale preference list. Now a list of locale is created and few locales are added. Using lookupTag() method, locales are searched and result is printed.
package com.tutorialspoint; import java.util.ArrayList; import java.util.List; import java.util.Locale; public class LocaleDemo { public static void main(String[] args) { String tags = Locale.ENGLISH.toLanguageTag() + "," + Locale.FRENCH.toLanguageTag(); List<Locale.LanguageRange> priorityList = Locale.LanguageRange.parse(tags); List<String> localeList = new ArrayList<>(); localeList.add("en"); localeList.add("jp"); String filteredTags = Locale.lookupTag(priorityList, localeList); System.out.println(filteredTags); } }
Output
Let us compile and run the above program, this will produce the following result −
en
Getting Best Matched Language Tag for en, en_CA, fr Locales Example
The following example shows the usage of Java Locale lookupTag() method. We're creating a different string of comma separated locales and using that string, we've created a locale preference list. Now a list of locale is created and few locales are added. Using lookupTag() method, locales are searched and result is printed.
package com.tutorialspoint; import java.util.ArrayList; import java.util.List; import java.util.Locale; public class LocaleDemo { public static void main(String[] args) { String tags = Locale.ENGLISH.toLanguageTag() + "," + Locale.FRENCH.toLanguageTag(); List<Locale.LanguageRange> priorityList = Locale.LanguageRange.parse(tags); List<String> localeList = new ArrayList<>(); localeList.add("en"); localeList.add("en_CA"); localeList.add("fr"); String filteredTags = Locale.lookupTag(priorityList, localeList); System.out.println(filteredTags); } }
Output
Let us compile and run the above program, this will produce the following result −
en
Getting Best Matched Language Tag for en, jp Locales Example
The following example shows the usage of Java Locale lookupTag() method. We're creating a string of comma separated locales and using that string, we've created a locale preference list. Now a list of locale is created and few locales are added. Using lookupTag() method, locales are searched and result is printed.
package com.tutorialspoint; import java.util.ArrayList; import java.util.List; import java.util.Locale; public class LocaleDemo { public static void main(String[] args) { String tags = Locale.ENGLISH.toLanguageTag() + "," + Locale.CANADA.toLanguageTag(); List<Locale.LanguageRange> priorityList = Locale.LanguageRange.parse(tags); List<String> localeList = new ArrayList<>(); localeList.add("en"); localeList.add("jp"); String filteredTags = Locale.lookupTag(priorityList, localeList); System.out.println(filteredTags); } }
Output
Let us compile and run the above program, this will produce the following result −
en
To Continue Learning Please Login