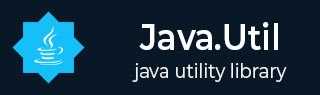
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java Locale filter() Method
Description
The Java Locale filter(List<Locale.LanguageRange> priorityList, Collection<Locale> locales) method returns a list of matching Locale instances using the filtering mechanism defined in RFC 4647. This is equivalent to filter(List, Collection, FilteringMode) when mode is Locale.FilteringMode.AUTOSELECT_FILTERING. This filter operation on the given locales ensures that only unique matching locale(s) are returned.
Declaration
Following is the declaration for java.util.Locale.filter() method
public static List<Locale> filter​(List<Locale.LanguageRange> priorityList, Collection<Locale> locales)
Parameters
priorityList − user's Language Priority List in which each language tag is sorted in descending order based on priority or weight.
locales − Locale instances used for matching.
Return Value
This method returns a list of Locale instances for matching language tags sorted in descending order based on priority or weight, or an empty list if nothing matches. The list is modifiable.
Exception
NullPointerException − if priorityList or locales is null
Java Locale filter(List<Locale.LanguageRange> priorityList, Collection<Locale> locales, Locale.FilteringMode mode) Method
Description
The Java Locale filter(List<Locale.LanguageRange> priorityList, Collection<Locale> locales, Locale.FilteringMode mode) method returns a list of matching Locale instances using the filtering mechanism defined in RFC 4647. This filter operation on the given locales ensures that only unique matching locale(s) are returned.
Declaration
Following is the declaration for java.util.Locale.filter() method
public static List<Locale> filter​(List<Locale.LanguageRange> priorityList, Collection<Locale> locales, Locale.FilteringMode mode)
Parameters
priorityList − user's Language Priority List in which each language tag is sorted in descending order based on priority or weight.
locales − Locale instances used for matching.
mode − filtering mode.
Return Value
This method returns a list of Locale instances for matching language tags sorted in descending order based on priority or weight, or an empty list if nothing matches. The list is modifiable.
Exception
NullPointerException − if priorityList or locales is null
IllegalArgumentException − if one or more extended language ranges are included in the given list when Locale.FilteringMode.REJECT_EXTENDED_RANGES is specified
Filtering a List of Locales Example
The following example shows the usage of Java Locale filter(List<Locale.LanguageRange>, Collection<Locale>) method. We're creating a string of comma separated locales and using that string, we've created a locale preference list. Now a list of locale is created and few locales are added. Using filter() method, locales are filtered and result is printed.
package com.tutorialspoint; import java.util.ArrayList; import java.util.List; import java.util.Locale; public class LocaleDemo { public static void main(String[] args) { String tags = Locale.ENGLISH.toLanguageTag() + "," + Locale.FRENCH.toLanguageTag(); List<Locale.LanguageRange> priorityList = Locale.LanguageRange.parse(tags); List<Locale> localeList = new ArrayList<>(); localeList.add(Locale.ENGLISH); localeList.add(Locale.JAPAN); List<Locale> filteredTags = Locale.filter(priorityList, localeList); System.out.println(filteredTags); } }
Output
Let us compile and run the above program, this will produce the following result −
[en]
Filtering a List of Locales Example
The following example shows the usage of Java Locale filter(List<Locale.LanguageRange>, Collection<Locale>) method. We're creating a different string of comma separated locales and using that string, we've created a locale preference list. Now a list of locale is created and few locales are added. Using filter() method, locales are filtered and result is printed.
package com.tutorialspoint; import java.util.ArrayList; import java.util.List; import java.util.Locale; public class LocaleDemo { public static void main(String[] args) { String tags = Locale.ENGLISH.toLanguageTag() + "," + Locale.FRENCH.toLanguageTag(); List<Locale.LanguageRange> priorityList = Locale.LanguageRange.parse(tags); List<Locale> localeList = new ArrayList<>(); localeList.add(Locale.ENGLISH); localeList.add(Locale.CANADA); localeList.add(Locale.FRENCH); List<Locale> filteredTags = Locale.filter(priorityList, localeList); System.out.println(filteredTags); } }
Output
Let us compile and run the above program, this will produce the following result −
[en, en_CA, fr]
Filtering a List of Locales With Filtering Mode Example
The following example shows the usage of Java Locale filter(List<Locale.LanguageRange>, Collection<Locale>,Locale.FilteringMode) method. We're creating a string of comma separated locales and using that string, we've created a locale preference list. Now a list of locale is created and few locales are added. Using filter() method, locales are filtered and result is printed.
package com.tutorialspoint; import java.util.ArrayList; import java.util.List; import java.util.Locale; public class LocaleDemo { public static void main(String[] args) { String tags = Locale.ENGLISH.toLanguageTag() + "," + Locale.FRENCH.toLanguageTag(); List<Locale.LanguageRange> priorityList = Locale.LanguageRange.parse(tags); List<Locale> localeList = new ArrayList<>(); localeList.add(Locale.ENGLISH); localeList.add(Locale.JAPAN); List<Locale> filteredTags = Locale.filter(priorityList, localeList,Locale.FilteringMode.AUTOSELECT_FILTERING); System.out.println(filteredTags); } }
Output
Let us compile and run the above program, this will produce the following result −
[en]
To Continue Learning Please Login