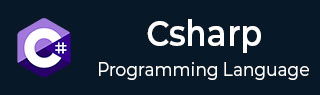
- C# Basic Tutorial
- C# - Home
- C# - Overview
- C# - Environment
- C# - Program Structure
- C# - Basic Syntax
- C# - Data Types
- C# - Type Conversion
- C# - Variables
- C# - Constants
- C# Operators
- C# - Operators
- C# - Arithmetic Operators
- C# - Assignment Operators
- C# - Relational Operators
- C# - Logical Operators
- C# - Bitwise Operators
- C# - Miscellaneous Operators
- C# - Operators Precedence
- C# Conditional Statements
- C# - Decision Making
- C# - If
- C# - If Else
- C# - Nested If
- C# - Switch
- C# - Nested Switch
- C# Control Statements
- C# - Loops
- C# - For Loop
- C# - While Loop
- C# - Do While Loop
- C# - Nested Loops
- C# - Break
- C# - Continue
- C# OOP & Data Handling
- C# - Encapsulation
- C# - Methods
- C# - Nullables
- C# - Arrays
- C# - Strings
- C# - Structure
- C# - Enums
- C# - Classes
- C# - Inheritance
- C# - Polymorphism
- C# - Operator Overloading
- C# - Interfaces
- C# - Namespaces
- C# - Preprocessor Directives
- C# - Regular Expressions
- C# - Exception Handling
- C# - File I/O
- C# Advanced Tutorial
- C# - Attributes
- C# - Reflection
- C# - Properties
- C# - Indexers
- C# - Delegates
- C# - Events
- C# - Collections
- C# - Generics
- C# - Anonymous Methods
- C# - Unsafe Codes
- C# - Multithreading
- C# Useful Resources
- C# - Questions and Answers
- C# - Cheatsheet
- C# - Quick Guide
- C# - Useful Resources
- C# - Discussion
C# - Continue Statement
C# continue Statement
The continue statement in C# works somewhat like the break statement. Instead of forcing termination, however, continue forces the next iteration of the loop to take place, skipping any code in between.
For the for loop, continue statement causes the conditional test and increment portions of the loop to execute. For the while and do...while loops, continue statement causes the program control passes to the conditional tests.
Syntax
The syntax for a continue statement in C# is as follows −
continue;
Flow Diagram

Using continue Statement with do while Loop
In this example, we use the continue statement to skip the iteration when a
becomes 15 −
using System; namespace Loops { class Program { static void Main(string[] args) { /* local variable definition */ int a = 10; /* do loop execution */ do { if (a == 15) { /* skip the iteration */ a = a + 1; continue; } Console.WriteLine("value of a: {0}", a); a++; } while (a < 20); Console.ReadLine(); } } }
Output
When the above code is compiled and executed, it produces the following result −
value of a: 10 value of a: 11 value of a: 12 value of a: 13 value of a: 14 value of a: 16 value of a: 17 value of a: 18 value of a: 19
Using continue Statement with for Loop
In this example, we use the continue statement to skip the current iteration and move to the next iteration of the loop −
using System; class ContinueExample { static void Main() { for (int i = 1; i <= 10; i++) { // Skip even numbers if (i % 2 == 0) { // Jump to the next iteration continue; } Console.WriteLine("Odd number: " + i); } Console.WriteLine("Loop completed."); } }
Output
The following is the output of the above code −
Odd number: 1 Odd number: 3 Odd number: 5 Odd number: 7 Odd number: 9 Loop completed.