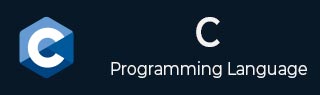
- C Programming Tutorial
- C - Home
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Tokens
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Constants
- C - Literals
- C - Escape sequences
- C - Storage Classes
- C - Operators
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- C - Loops
- C - While loop
- C - For loop
- C - Do...while loop
- C - Nested loop
- C - Infinite loop
- C - Break Statement
- C - Continue Statement
- C - goto Statement
- C - Functions
- C - Main Functions
- C - Return Statement
- C - Recursion
- C - Scope Rules
- C - Arrays
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- C - Pointers
- C - Pointer Arithmetics
- C - Passing Pointers to Functions
- C - Strings
- C - Array of Strings
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Pointers to Structures
- C - Self-Referential Structures
- C - Nested Structures
- C - Unions
- C - Bit Fields
- C - Typedef
- C - Input & Output
- C - File I/O
- C - Preprocessors
- C - Header Files
- C - Type Casting
- C - Error Handling
- C - Variable Arguments
- C - Memory Management
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Useful Resources
- C - Discussion
Break Statement in C
The break statement in C is used in two different contexts. In switch-case, break is placed as the last statement of each case block. The break statement may also be employed in the body of any of the loop constructs (while, do–while as well as for loops).
When used inside a loop, break causes the loop to be terminated. In the switch-case statement, break takes the control out of the switch scope after executing the corresponding case block.
Flowchart of Break Statement in C
The flowchart of break in loop is as follows −
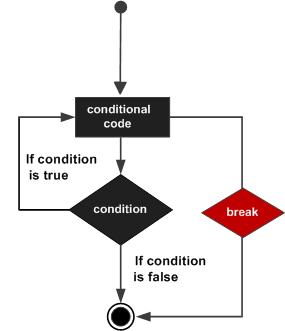
The following flowchart shows how to use break in switch-case −
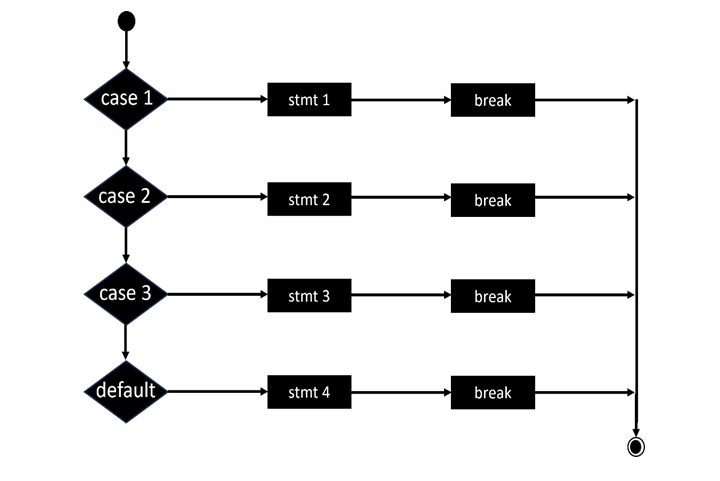
In both the scenarios, break causes the control to be taken out of the current scope.
Using Break Statements in While Loops
The break statement is never used unconditionally. It always appears in the True part of an if statement. Otherwise, the loop will terminate in the middle of the first iteration itself.
while(condition1){ . . . . . . if(condition2) break; . . . . . . }
Example
The following program checks if a given number is prime or not. A prime number is not divisible by any other number except itself and 1.
The while loop increments the divisor by 1 and tries to check if it is divisible. If found divisible, the while loop is terminated.
#include <stdio.h> /*break in while loop*/ int main () { int i = 2; int x = 121; printf("x: %d\n", x); while (i < x/2){ if (x % i == 0) break; i++; } if (i >= x/2) printf("%d is prime", x); else printf("%d is not prime", x); return 0; }
Output
On executing this code, you will get the following output −
x: 121 121 is not prime
Now, change the value of "x" to 25 and run the code again. It will produce the following output −
x: 25 25 is not prime
Using Break Statements in For Loops
You can use a break statement inside a for loop as well. Usually, a for loop is designed to perform a certain number of iterations. However, sometimes it may be required to abandon the loop if a certain condition is reached.
The usage of break in for loop is as follows −
for (init; condition; increment) { . . . if (condition) break; . . . }
Example
The following program prints the characters from a given string before a vowel (a, e, I, or u) is detected.
#include <stdio.h> #include <string.h> int main () { char string[] = "Rhythmic"; int len = strlen(string); int i; for (i = 0; i < len; i++){ if (string[i] == 'a' || string[i] == 'e' || string[i] == 'i' || string[i] == 'o' || string[i] == 'u') break; printf("%c\n", string[i]); } return 0; }
Output
Run the code and check its output −
R h y t h m
If break appears in an inner loop of a nested loop construct, it abandons the inner loop and continues the iteration of the outer loop body. For the next iteration, it enters the inner loop again, which may be broken again if the condition is found to be true.
Example
In the following program, two nested loops are employed to obtain a list of all the prime numbers between 1 to 30. The inner loop breaks out when a number is found to be divisible, setting the flag to 1. After the inner loop, the value of flag is checked. If it is "0", the number is a prime number.
#include <stdio.h> int main(){ int i, num, n, flag; printf("The prime numbers in between the range 1 to 30:\n"); for(num = 2; num <= 30; num++){ flag = 0; for(i = 2; i <= num/2; i++){ if(num % i == 0){ flag++; break; } } if(flag == 0) printf("%d is prime\n",num); } return 0; }
Output
2 is prime 3 is prime 5 is prime 7 is prime 11 is prime 13 is prime 17 is prime 19 is prime 23 is prime 29 is prime
Using Break Statement in an Infinite Loop
An infinite loop is rarely created intentionally. However, in some cases, you may start an infinite loop and break from it when a certain condition is reached.
Example
In the following program, an infinite for loop is used. On each iteration, a random number between 1 to 100 is generated till a number that is divisible by 5 is obtained.
#include <stdio.h> #include <stdlib.h> #include <time.h> int main(){ int i, num; printf ("Program to get the random number from 1 to 100: \n"); srand(time(NULL)); for (; ; ){ num = rand() % 100 + 1; // random number between 1 to 100 printf (" %d\n", num); if (num%5 == 0) break; } }
Output
On running this code, you will get an output like the one shown here −
Program to get the random number from 1 to 100: 6 56 42 90
Using Break Statements in Switch-Case
To transfer the control out of the switch scope, every case block ends with a break statement. If not, the program falls through all the case blocks, which is not desired.
Example
In the following code, a series of if-else statements print three different greeting messages based on the value of a "ch" variable ("m", "a" or "e" for morning, afternoon or evening).
#include <stdio.h> int main(){ /* local variable definition */ char ch = 'm'; printf("Time code: %c\n\n", ch); switch(ch) { case 'm': printf("Good Morning \n"); break; case 'a': printf("Good Afternoon \n"); break; case 'e': printf("Good Evening \n"); break; default: printf("Hello"); } }
Output
Here, the break statement breaks the program execution after checking the first case.
Time code: m Good Morning
Now, comment the break statements and run the code again. You will now get the following output −
Time code: m Good Morning Good Afternoon Good Evening Hello