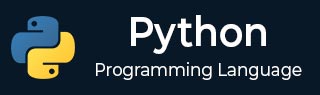
- Python Basics
- Python - Home
- Python - Overview
- Python - History
- Python - Features
- Python vs C++
- Python - Hello World Program
- Python - Application Areas
- Python - Interpreter
- Python - Environment Setup
- Python - Virtual Environment
- Python - Basic Syntax
- Python - Variables
- Python - Data Types
- Python - Type Casting
- Python - Unicode System
- Python - Literals
- Python - Operators
- Python - Arithmetic Operators
- Python - Comparison Operators
- Python - Assignment Operators
- Python - Logical Operators
- Python - Bitwise Operators
- Python - Membership Operators
- Python - Identity Operators
- Python - Operator Precedence
- Python - Comments
- Python - User Input
- Python - Numbers
- Python - Booleans
- Python Control Statements
- Python - Control Flow
- Python - Decision Making
- Python - If Statement
- Python - If else
- Python - Nested If
- Python - Match-Case Statement
- Python - Loops
- Python - for Loops
- Python - for-else Loops
- Python - While Loops
- Python - break Statement
- Python - continue Statement
- Python - pass Statement
- Python - Nested Loops
- Python Functions & Modules
- Python - Functions
- Python - Default Arguments
- Python - Keyword Arguments
- Python - Keyword-Only Arguments
- Python - Positional Arguments
- Python - Positional-Only Arguments
- Python - Arbitrary Arguments
- Python - Variables Scope
- Python - Function Annotations
- Python - Modules
- Python - Built in Functions
- Python Strings
- Python - Strings
- Python - Slicing Strings
- Python - Modify Strings
- Python - String Concatenation
- Python - String Formatting
- Python - Escape Characters
- Python - String Methods
- Python - String Exercises
- Python Lists
- Python - Lists
- Python - Access List Items
- Python - Change List Items
- Python - Add List Items
- Python - Remove List Items
- Python - Loop Lists
- Python - List Comprehension
- Python - Sort Lists
- Python - Copy Lists
- Python - Join Lists
- Python - List Methods
- Python - List Exercises
- Python Tuples
- Python - Tuples
- Python - Access Tuple Items
- Python - Update Tuples
- Python - Unpack Tuples
- Python - Loop Tuples
- Python - Join Tuples
- Python - Tuple Methods
- Python - Tuple Exercises
- Python Sets
- Python - Sets
- Python - Access Set Items
- Python - Add Set Items
- Python - Remove Set Items
- Python - Loop Sets
- Python - Join Sets
- Python - Copy Sets
- Python - Set Operators
- Python - Set Methods
- Python - Set Exercises
- Python Dictionaries
- Python - Dictionaries
- Python - Access Dictionary Items
- Python - Change Dictionary Items
- Python - Add Dictionary Items
- Python - Remove Dictionary Items
- Python - Dictionary View Objects
- Python - Loop Dictionaries
- Python - Copy Dictionaries
- Python - Nested Dictionaries
- Python - Dictionary Methods
- Python - Dictionary Exercises
- Python Arrays
- Python - Arrays
- Python - Access Array Items
- Python - Add Array Items
- Python - Remove Array Items
- Python - Loop Arrays
- Python - Copy Arrays
- Python - Reverse Arrays
- Python - Sort Arrays
- Python - Join Arrays
- Python - Array Methods
- Python - Array Exercises
- Python File Handling
- Python - File Handling
- Python - Write to File
- Python - Read Files
- Python - Renaming and Deleting Files
- Python - Directories
- Python - File Methods
- Python - OS File/Directory Methods
- Object Oriented Programming
- Python - OOPs Concepts
- Python - Object & Classes
- Python - Class Attributes
- Python - Class Methods
- Python - Static Methods
- Python - Constructors
- Python - Access Modifiers
- Python - Inheritance
- Python - Polymorphism
- Python - Method Overriding
- Python - Method Overloading
- Python - Dynamic Binding
- Python - Dynamic Typing
- Python - Abstraction
- Python - Encapsulation
- Python - Interfaces
- Python - Packages
- Python - Inner Classes
- Python - Anonymous Class and Objects
- Python - Singleton Class
- Python - Wrapper Classes
- Python - Enums
- Python - Reflection
- Python Errors & Exceptions
- Python - Syntax Errors
- Python - Exceptions
- Python - try-except Block
- Python - try-finally Block
- Python - Raising Exceptions
- Python - Exception Chaining
- Python - Nested try Block
- Python - User-defined Exception
- Python - Logging
- Python - Assertions
- Python - Built-in Exceptions
- Python Multithreading
- Python - Multithreading
- Python - Thread Life Cycle
- Python - Creating a Thread
- Python - Starting a Thread
- Python - Joining Threads
- Python - Naming Thread
- Python - Thread Scheduling
- Python - Thread Pools
- Python - Main Thread
- Python - Thread Priority
- Python - Daemon Threads
- Python - Synchronizing Threads
- Python Synchronization
- Python - Inter-thread Communication
- Python - Thread Deadlock
- Python - Interrupting a Thread
- Python Networking
- Python - Networking
- Python - Socket Programming
- Python - URL Processing
- Python - Generics
- Python Libraries
- NumPy Tutorial
- Pandas Tutorial
- SciPy Tutorial
- Matplotlib Tutorial
- Django Tutorial
- OpenCV Tutorial
- Python Miscellenous
- Python - Date & Time
- Python - Maths
- Python - Iterators
- Python - Generators
- Python - Closures
- Python - Decorators
- Python - Recursion
- Python - Reg Expressions
- Python - PIP
- Python - Database Access
- Python - Weak References
- Python - Serialization
- Python - Templating
- Python - Output Formatting
- Python - Performance Measurement
- Python - Data Compression
- Python - CGI Programming
- Python - XML Processing
- Python - GUI Programming
- Python - Command-Line Arguments
- Python - Docstrings
- Python - JSON
- Python - Sending Email
- Python - Further Extensions
- Python - Tools/Utilities
- Python - GUIs
- Python Useful Resources
- Python Compiler
- NumPy Compiler
- Matplotlib Compiler
- SciPy Compiler
- Python - Programming Examples
- Python - Quick Guide
- Python - Useful Resources
- Python - Discussion
Python Online Quiz
Following quiz provides Multiple Choice Questions (MCQs) related to Python. You will have to read all the given answers and click over the correct answer. If you are not sure about the answer then you can check the answer using Show Answer button. You can use Next Quiz button to check new set of questions in the quiz.
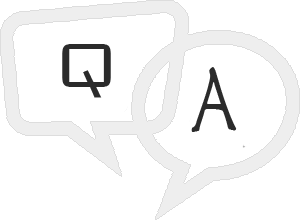
Q 1 - What is the output for −
S = [['him', 'sell'], [90, 28, 43]]
S[0][1][1]
Answer : A
Explanation
List can contain other list values.
So, in this question S[0] gives ['him', 'sell'], S[0][1] gives ‘sell' and S[0][1][1] gives ‘e'.
Remember, the index in python starts with ‘0'.
Q 2 - What is output of following code −
a = (1, 2) a[0] +=1
Answer : C
Explanation
TypeError − ‘tuple' object does not support item assignment because a tuple is immutable.
Q 3 - How can we generate random numbers in python using methods?
Answer : D
Explanation
To generate random numbers we import random module and in that module we have these methods/functions.
uniform(x,y) returns a floating number in the range [x,y] random() returns a floating point number in the range [0, 1].
randint(x,y) returns a random integer number in the range [x, y].
Q 4 - What is output of following code −
def func(n): if(n==1): return 1; else: return(n+func(n-1)) print(func(4))
Answer : B
Q 5 - What is the output of the code?
def f(): global z print('z is: ', z) z=50 print('new value of global z is: ', z) f() print('Value of z is: ', z)
new value of global z is: 100
value of z is 100
new value of global z is: 100
value of z is 50
new value of global z is: 50
value of z is 100
new value of global z is: 50
value of z is 50
Answer : D
Explanation
Here in the above code ‘global' keyword is used to state that ‘z' is global variable. So when we assign a value to z in the function then the new assigned value is reflected whenever we use the value of ‘z' in the main block or outside the function.
Q 6 - Guess the output −
def main(): try: func() print(''print this after function call'') except ZeroDivisionError: print('Divided By Zero! Not Possible! ') except: print('Its an Exception!') def func(): print(1/0) main()
B - ‘Divided By Zero! Not possible!'
C - ‘print this after function call' followed by ‘Divided By Zero! Not Possible!'
D - ‘print this after function call' followed by ‘Its an Exception!'
Answer : B
Explanation
The function ‘func' will not run because it contains an exception. So in try and expect block. The function called under try will not run and will move to except block which defines the type of exception present in the function ‘func'. Thus block of statements present in except ZeroDivisionError is printed.
Q 7 - Discuss the outcome of the code?
def func1(n): if(n==0): return 0 else: return(n+func1(n-1)) def func2(n, result): if(n==0): return(result) else: return(func2(n-1, n+result)) print(func1(4)) print(func2(4,0))
B - Func1 and Func2 are tail recursions.
Answer : B
Explanation
A function call is said to be tail recursive if there is nothing to do after the function returns except return its value.
Q 8 - Choose the correct syntax for reading from a text file stored in ‘‘c:\scores.txt'' ?
A - Infile = open(''c:\scores.txt'',''r'')
B - Infile = open(file=''c:\\\scores.txt'', ''r'')
Answer : D
Explanation
You need to define the format in which you want to open the file. Proper path has to be declared by the user for the interpreter to reach the destination of the file.
Q 9 - Select the code for the following output?

A - Canvas.showinfo(''showinfo'', ''Programming'')
B - Tkinter.showinfo(''showinfo'' , ''Programming'')
C - Tkinter.messagebox.showinfo(''Programming'')
D - Tkinter.messagebox.showinfo(''showinfo'' , ''Programming'')
Answer : D
Answer : C
Explanation
Because Identifiers start from letter A to Z or a to z or an underscore (_) followed by more letters or zero or underscores and digits (0 to 9). Python does not allow @ or $ or % within the identifier.
To Continue Learning Please Login