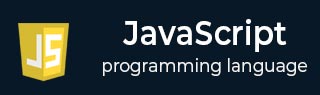
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - TypedArray reduceRight() Method
The JavaScript TypedArray reduceRight() method executes a callback function against an accumulator and each value of the current typed array from right to left to reduce it to a single value.
If no initial value is passed to this method, it will use the last element of the typed array as an initial value and skip. If you call the reduceRight() on an empty typed array, it will return a 'TypeError' exception.
Both reduceRight() and reduce() methods are very similar to each other the only difference is the direction of iteration. The reduceRight() method iterates over the typed array from right to left, whereas the reduce() method iterates from left to right.
Syntax
Following is the syntax of JavaScript TypedArray reduceRight() method −
reduceRight(callbackFn, initialValue)
Parameters
This method accepts two parameters named 'callbackFn' and 'initialValue', which are described below −
callbackFn − A user-provided function to execute on each element of the typed array.
This callbackFn function also accepts four parameters, namely 'accumulator', 'currentValue', 'currentIndex', and 'array'. Here's the description for each parameter −
accumulator − This parameter represents the accumulated value resulting from previous iterations. It starts with the initial value (either provided explicitly or the first element of the array) and gets updated with each iteration.
currentValue − The value of the current element in the typed array. If the initialValue is specified, its value will be the last element, if not its value will be the second to last element.
currentIndex − The index position of the currentValue in the typed array.
array − The typed array on which the reduceRight() method gets called.
initialValue − The value to which the accumulator parameter is initialized when the first time the callback function is called.
Return value
This method returns a value that results from the reduction.
Examples
Example 1
In the following example, we are using the JavaScript TypedArray reduceRight() method to execute the user-provided callback function named RighttoLeft() on each element of the typed array from right to left. This function returns this typed array element [10, 20, 30, 40, 50].
<html> <head> <title>JavaScript TypedArray reduceRight() Method</title> </head> <body> <script> //function function RighttoLeft(accumulator, currentValue){ return `${accumulator}, ${currentValue}`; } const T_array = new Uint16Array([10, 20, 30, 40, 50]); document.write("Typed array: ", T_array); //using the reduceRight() method document.write("<br>The reversed typed array: ", T_array.reduceRight(RighttoLeft)); </script> </body> </html>
Output
This program will return the typed array elements [10, 20, 30, 40, 50] from right to left as −
Typed array: 10, 20, 30, 40, 50 The reversed typed array: 50, 40, 30, 20, 10
Example 2
The product of all elements within a typed array.
In this example, we are using the JavaScript TypedArray reduceRight() method to execute the user-provided function named multi() on each element of this typed array [1, 2, 3, 4, 5] from right to left. The multi() function multiplies all the elements within the current typed array.
<html> <head> <title>JavaScript TypedArray reduceRight() Method</title> </head> <body> <script> //function function multi(accumulator, currentValue){ return accumulator * currentValue; } const T_array = new Uint16Array([1, 2, 3, 4, 5]); document.write("Typed array: ", T_array); //using the reduceRight() method document.write("<br>The product of typed array elements: ", T_array.reduceRight(multi)); </script> </body> </html>
Output
The above program returns the product of typed array elements as 120.
Typed array: 1,2,3,4,5 The product of typed array elements: 120
Example 3
If we pass the initialValue parameter to this method, the reduceRight() method also executes the user-provided callback function on it and returns a single value as a result.
In the following example, we use the JavaScript TypedArray reduceRight() method to execute the user-provided callback function named sum() on each element of the typed array [10, 15, 20, 25, 30] from right to left, including the passed initialValue parameter value 15. The sum() function accumulates the total sum of all elements within the current typed array.
<html> <head> <title>JavaScript TypedArray reduceRight() Method</title> </head> <body> <script> const T_array = new Uint16Array([10, 20, 30, 40, 50]); document.write("Typed array: ", T_array); const initialValue = 10; document.write("<br>Initial value: ", initialValue); let sum = T_array.reduce(function(accumulator, currentValue){ return accumulator + currentValue; }, initialValue); document.write("<br>The sum of typed array elements(including initial value): ", sum); </script> </body> </html>
Output
After executing the above program, it will return the sum of all elements within the typed array including the initial value −
Typed array: 10,15,20,25,30 Initial value: 10 The sum of typed array elements(including initial value): 160
Example 4
If the current typed array does not contain any element (no initial value available), the reduceRight() method will throw a "TypeError" exception.
In the given example below, we use the JavaScript TypedArray reduceRight() method to execute the user-provided reducer callback function named "ArrowFunction" on each element from right to left of this empty typed array ([]).
<html> <head> <title>JavaScript TypedArray reduceRight() Method</title> </head> <body> <script> const T_array = new Uint16Array([]);//empty typed array document.write("Length of the typed array: ", T_array.length); //using the reduce method try { T_array.reduce((a, b)=> a + b); } catch (error) { document.write("<br>", error); } </script> </body> </html>
Output
Once the above program is executed, it will throw a 'TypeError' exception.
Length of the typed array: 0 TypeError: Reduce of empty array with no initial value
To Continue Learning Please Login