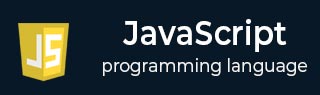
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - TypedArray reduce() Method
The JavaScript TypedArray reduce() method executes the "reducer" callback function provided by the user on each element of the typed array. It returns a single value as a result obtained from executing the callback function over the entire typed array.
It accepts an optional parameter named 'initialValue'. If we do not pass this parameter to the method, it will consider the arr[0] value as the initial value. Additionally, it will execute the callback function on the passed initialValue parameter.
Note − If the current TypedArray is empty or doesn't contain any initial value, this method will throw a 'TypeError' exception.
Syntax
Following is the syntax of JavaScript TypedArray reduce() method −
reduce(callbackFn, initialValue)
Parameters
This method accepts two parameters named 'callbackFn' and 'initialValue', which are described below −
callbackFn − A user-provided function to execute on each element of the typed array.
This callbackFn function also accepts four parameters, namely 'accumulator', 'currentValue', 'currentIndex', and 'array'. Here's the description for each parameter:
accumulator − This parameter represents the accumulated value resulting from previous iterations. It starts with the initial value (either provided explicitly or the first element of the array) and gets updated with each iteration.
currentValue − The value of the current element in typed array. If the initialValue is specified, its value will be arr[0], if not it's value will be arr[1].
currentIndex − The index position of the currentValue in the typed array.
array − The typed array on which the reduce() method gets called.
initialValue − The value to which the accumulator parameter is initialized when the first time the callback function is called.
Return value
This method returns a single value that results from executing the "reducer" callback function to completion over the entire typed array.
Examples
Example 1
The product of all elements within a typed array.
In the following example, we are using the JavaScript TypedArray reduce() method to execute the user-provided function named multi() on each element of this typed array [1, 2, 3, 4, 5]. The multi() function multiplies all the elements within the current typed array.
<html> <head> <title>JavaScript TypedArray reduce() Method</title> </head> <body> <script> //function function multi(accumulator, currentValue){ return accumulator * currentValue; } const T_array = new Uint16Array([1, 2, 3, 4, 5]); document.write("Typed array: ", T_array); //using the reduce() method document.write("<br>The product of typed array elements: ", T_array.reduce(multi)); </script> </body> </html>
Output
The above program returns the product of typed array elements as 120.
Typed array: 1,2,3,4,5 The product of typed array elements: 120
Example 2
If we pass the initialValue parameter to this method, the reduce() method also executes the user-provided callback function on it and returns a single value as a result.
In the following example, we use the JavaScript TypedArray reduce() method to execute the user-provided callback function named sum() on each element of the typed array [10, 20, 30, 40, 50], including the passed initialValue parameter value 10. The sum() function accumulates the total sum of all elements within the current typed array.
<html> <head> <title>JavaScript TypedArray reduce() Method</title> </head> <body> <script> const T_array = new Uint16Array([10, 20, 30, 40, 50]); document.write("Typed array: ", T_array); const initialValue = 10; document.write("<br>Initial value: ", initialValue); let sum = T_array.reduce(function(accumulator, currentValue){ return accumulator + currentValue; }, initialValue); document.write("<br>The sum of typed array elements(including initial value): ", sum); </script> </body> </html>
Output
After executing the above program, it will return the sum of all elements within the typed array including the initial value −
Typed array: 10,20,30,40,50 Initial value: 10 The sum of typed array elements(including initial value): 160
Example 3
If the current typed array does not contain any element(no initial value available), the reduce() method will throw a "TypeError" exception.
In the given example below, we use the JavaScript TypedArray reduce() method to execute the user-provided reducer callback function named "ArrowFunction" on each element of this empty typed array ([]).
<html> <head> <title>JavaScript TypedArray reduce() Method</title> </head> <body> <script> const T_array = new Uint16Array([]);//empty typed array document.write("Length of the typed array: ", T_array.length); //using the reduce method try { T_array.reduce((a, b)=> a + b); } catch (error) { document.write("<br>", error); } </script> </body> </html>
Output
Once the above program is executed, it will throw a 'TypeError' exception.
Length of the typed array: 0 TypeError: Reduce of empty array with no initial value
To Continue Learning Please Login