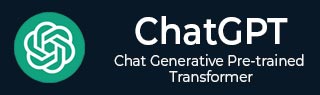
- ChatGPT Tutorial
- ChatGPT - Home
- ChatGPT - Fundamentals
- ChatGPT - Getting Started
- ChatGPT - How It Works
- ChatGPT - Prompts
- ChatGPT - Competitors
- ChatGPT - For Content Creation
- ChatGPT - For Marketing
- ChatGPT - For Job Seekers
- ChatGPT - For Code Writing
- ChatGPT - For SEO
- ChatGPT - Machine Learning
- ChatGPT - Generative AI
- ChatGPT - Build a Chatbot
- ChatGPT - Plugin
- ChatGPT Useful Resources
- ChatGPT - Quick Guide
- ChatGPT - Useful Resources
- ChatGPT - Discussion
ChatGPT – For Content Creation
Since its launch, ChatGPT has captured the attention of content creators faster than expected. In this chapter, we will see various ways to use ChatGPT for content creation. Along with that, we will also see Python implementation using OpenAI API.
Get an API Key from OpenAI
First of all, you'll need to sign up on the OpenAI platform and obtain an API key. Once you have your API key, you can install the OpenAI Python library as follows −
pip install openai
Now, you're ready to infuse your content creation projects with the creative capabilities of ChatGPT.
Generating Text Using ChatGPT
In its capacity as a language model, ChatGPT excels at crafting text in accordance with user prompts.
For example, you can use ChatGPT to generate a story as below −
import openai # Set your OpenAI API key openai.api_key = 'your-api-key-goes-here' # Define a prompt for text generation prompt = "Write a short story about a detective solving a mysterious case." # Specify the OpenAI engine and make a request response = openai.Completion.create( engine=" gpt-3.5-turbo-instruct", prompt=prompt, max_tokens=100 ) # Extract the generated text from the API response generated_text = response['choices'][0]['text'] # Print or use the generated text as needed print(generated_text)
Note − Replace "your-api-key-goes-here" with your actual OpenAI API key. The above example prompts the model to generate a short story about a detective, and you can customize the prompt and other parameters based on your specific use case.
In this case, we got the following output −
Detective Mark Reynolds had been on the force for over a decade. He had seen his share of mysteries and solved his fair share of cases. But the one he was currently working on was unlike any he had encountered before.
Note that the system may produce a different response on your system when you use the same code with your OpenAI key.
Generating Video Scripts Using ChatGPT
As we know generating video content requires scripting and ChatGPT can help you in the creation of video scripts. You can utilize the generated text as a foundation for initiating your video content creation journey. Let’s check out the below example −
import openai # Set your OpenAI API key openai.api_key = 'your-api-key-goes-here' # Define a prompt for generating a video script prompt = "Create a script for a promotional video showcasing our new AI product." # Specify the OpenAI engine and make a request response = openai.Completion.create( engine="gpt-3.5-turbo-instruct", prompt=prompt, max_tokens=100 ) # Extract the generated script from the API response generated_script = response['choices'][0]['text'] # Print or use the generated script as needed print(generated_script)
In this case, we got the following output −
[Opening shot of a modern office space with employees working at their desks] Voiceover: Are you tired of mundane tasks taking up valuable time at work? Do you wish there was a way to streamline your workflow and increase productivity? [Cut to employees looking stressed and overwhelmed] Voiceover: Well, look no further. Our company is proud to introduce our latest innovation – our revolutionary AI product. [Cut to a sleek and futuristic AI device on a desk]
Music Composition Using ChatGPT
ChatGPT can be used for music composition by providing it with a musical prompt or request. The generated musical ideas or lyrics can be then used as inspiration for your compositions.
Here's a simple example that demonstrates how ChatGPT can generate a short piano melody based on the given prompt −
import openai # Set your OpenAI API key openai.api_key = 'your-api-key-goes-here' # Define a prompt for music composition prompt = "Compose a short piano melody in the key of C major." # Specify the OpenAI engine and make a request response = openai.Completion.create( engine="gpt-3.5-turbo-instruct", prompt=prompt, max_tokens=300 ) # Extract the generated music composition from the API response generated_music = response['choices'][0]['text'] # Print or use the generated music as needed print(generated_music)
In this case, we got the following output −
Here is a simple piano melody in the key of C major: C D E F G A G F E D C B A C The melody begins and ends on C, the tonic note of the C major scale. It then moves up and down the scale, primarily using steps and occasionally skipping a note up or down. This gives the melody a smooth and pleasant flow.
Note that you can customize the prompt to guide the style, genre, or specific elements of the music you want to create.
Generating Interactive Content Using ChatGPT
You can also use ChatGPT to generate dynamic dialogues, quizzes, or choose-your-own-adventure narratives. Let’s see an example where we are using ChatGPT to generate dynamic dialogues for a school play on the topic "Robotics and society".
import openai # Set your OpenAI API key openai.api_key = 'your-api-key-goes-here' # Define a prompt for generating dialogues prompt = "Write dynamic dialogues for a school play on the topic 'Robotics and society'." # Specify the OpenAI engine and make a request response = openai.Completion.create( engine="gpt-3.5-turbo-instruct", prompt=prompt, max_tokens=100 ) # Extract the generated dialogues from the API response generated_dialogues = response['choices'][0]['text'] # Print or use the generated dialogues as needed print(generated_dialogues)
The following generated text can serve as a starting point for creating engaging and dynamic dialogues for the play −
(Scene opens with a group of students gathered around a table, discussing about a robotics project) Student 1: Okay everyone, let's finalize our project idea for the robotics competition. Student 2: How about a robot that assists elderly people in their daily tasks? Student 3: That's a great idea, but I think we can do something more impactful for society.
Content Enhancement Using ChatGPT
ChatGPT can be utilized for creative suggestions, enhancements, or even summarization by providing it with specific instructions to improve or expand upon existing content. In the below example the model is asked to enhance the provided content −
import openai # Set your OpenAI API key openai.api_key = 'your-api-key-goes-here' # Define content that needs enhancement input_content = "The importance of renewable energy sources cannot be overstated. They play a crucial role in reducing our reliance on non-renewable resources." # Create a prompt for content enhancement prompt = f"Enhance the following text:\n\n{input_content}" # Specify the OpenAI engine and make a request response = openai.Completion.create( engine="gpt-3.5-turbo-instruct", prompt=prompt, max_tokens=100 ) # Extract the enhanced content from the API response enhanced_content = response['choices'][0]['text'] # Print or use the enhanced content as needed print(enhanced_content)
The model will give the enhanced content as needed −
The significance of renewable energy sources cannot be overstated. In today's world, where concerns about climate change and resource depletion are at an all-time high, these sources of energy have become essential. They not only offer a cleaner and more sustainable alternative to traditional, non-renewable resources, but also play a crucial role in reducing our carbon footprint and mitigating the adverse effects of global warming. Renewable energy sources, such as solar, wind, hydro, geothermal, and biomass, are constantly replenished and therefore do not deplete as traditional fossil fuels do. This makes them highly valuable in promoting a more secure and sustainable energy future.
Keep in mind that the result depends on the model's understanding and creativity, and you may need to iterate or experiment to achieve the desired level of enhancement.
Content Personalization Using ChatGPT
ChatGPT can be used for content personalization by tailoring text to specific individuals or audiences. The following example shows how you can utilize user data to personalize generated text, making it more relevant and engaging −
import openai # Set your OpenAI API key openai.api_key = 'your-api-key-goes-here' # User-specific information user_name = "Gaurav" user_interest = "ChatGPT" # Define a prompt for personalized content prompt = f"Generate personalized content for {user_name}. Focus on {user_interest}." # Specify the OpenAI engine and make a request response = openai.Completion.create( engine="gpt-3.5-turbo-instruct", prompt=prompt, max_tokens=200 ) # Extract the personalized content from the API response personalized_content = response['choices'][0]['text'] # Print or use the personalized content as needed print(personalized_content)
The model will give the personalized content as below −
Hello Gaurav! Have you heard about ChatGPT? ChatGPT is an innovative chatbot that uses advanced artificial intelligence to have human-like conversations with you. This means that you can talk to ChatGPT just like you would talk to a friend or a real person. One of the most amazing things about ChatGPT is its ability to understand natural language. This means that you don't have to use specific keywords or phrases to communicate with it. You can simply chat with ChatGPT in your own words, and it will understand and respond to you just like a human would.
Conclusion
In this chapter, we learned how ChatGPT can help make text, create video scripts, compose music, and even make interactive content better. We demonstrated how ChatGPT can be like a helpful friend in different creative tasks.
To Continue Learning Please Login