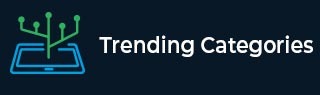
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
XNOR of two numbers
XNOR(Exclusive-NOR) gate is a digital logic gate that takes two inputs and gives one output. Its function is the logical complement of the Exclusive-OR (XOR) gate. The output is TRUE if both inputs are the same and FALSE if the inputs are different. The truth table of the XNOR gate is given below.
A | B | Output |
---|---|---|
1 | 1 | 1 |
1 | 0 | 0 |
0 | 1 | 0 |
0 | 0 | 1 |
Problem Statement
Given two numbers x and y. Find the XNOR of the two numbers.
Sample Example 1
Input: x = 12, y = 5
Output: 6
Explanation
(12)10 = (1100)2 (5)10 = (101)2 XNOR = (110)2 = (6)10
Sampe Example 2
Input: x = 16, y = 16
Output: 31
Explanation
(16)10 = (10000)2 (16)10 = (10000)2 XNOR = (11111)2 = (31)10
Approach 1: Brute Force Approach
The brute force approach will be to check each bit of both numbers and compare if they are the same or not. If they are the same, put 1 else 0.
Pseudocode
procedure xnor (x, y) if x > y then swap(x,y) end if if x == 0 and y == 0 then ans = 1 end if while x x_rem = x & 1 y_rem = y & 1 if x_rem == y_rem then ans = ans | (1 << count) end if count = count + 1 x = x >> 1 y = y >> 1 end procedure
Example: C++ Implementation
In the following program, check if bits of both x and y are same and then set bits in the answer.
#include <bits/stdc++.h> using namespace std; // Function to swap values of two variables void swap(int *a, int *b){ int temp = *a; *a = *b; *b = temp; } // Function to find the XNOR of two numbers int xnor(int x, int y){ // Placing the lower number in variable x if (x > y){ swap(x, y); } // Base Condition if (x == 0 && y == 0){ return 1; } // Cnt for counting the bit position Ans stores ans sets the bits of XNOR operation int cnt = 0, ans = 0; // executing loop for all the set bits in the lower number while (x){ // Gets the last bit of x and y int x_rem = x & 1, y_rem = y & 1; // If last bits of x and y are same if (x_rem == y_rem){ ans |= (1 << cnt); } // Increase counter for bit position and right shift both x and y cnt++; x = x >> 1; y = y >> 1; } return ans; } int main(){ int x = 10, y = 11; cout << "XNOR of " << x << " and " << y << " = " << xnor(x, y); return 0; }
Output
XNOR of 10 and 11 = 14
Time Complexity: O(logn) as traversal is done on all the bits of number which are logn.
Space Complexity: O(1)
Approach 2
XNOR is the inverse of XOR operation and it’s truth table is also the inverse of XOR table. Thus, toggling the bits of the higher number i.e. setting 1 to 0 and 0 to 1 and then XORing with the lower number will yield XNOR number.
Sampe Example 1
Input: x = 12, y = 5
Output: 6
Explanation
(12)10 = (1100)2 (5)10 = (101)2 Toggled bits of 12 = 0011 0011 ^ 101 = 0110
Sampe Example 2
Input: x = 12, y = 31
Output: 12
Explanation
(12)10 = (1100)2 (31)10 = (11111)2 Toggled bits of 31 = 00000 00000 ^ 1100 = 01100
Pseudocode
procedure toggle (n) temp = 1 while temp <= n n = n ^ temp temp = temp << 1 end procedure procedure xnor (x, y) max_num = max(x,y) min_num = min(x,y) toggle (max_num) ans = max_num ^ min_num end procedure
Example: C++ Implementation
In the following program, all the bits of higher number are toggled and then XORed with the lower number.
#include <bits/stdc++.h> using namespace std; // Function to toggle all bits of a number void toggle(int &num){ int temp = 1; // Execute loop until set bit of temp cross MST of num while (temp <= num){ // Toggle bit of num corresponding to set bit in temp num ^= temp; // Move set bit of temp to left temp <<= 1; } } // Function to find the XNOR of two numbers int xnor(int x, int y){ // Finding max and min number int max_num = max(x, y), min_num = min(x, y); // Togglinf the max number toggle(max_num); // XORing toggled max num and min num return max_num ^ min_num; } int main(){ int x = 5, y = 15; cout << "XNOR of " << x << " and " << y << " = " << xnor(x, y); return 0; }
Output
XNOR of 5 and 15 = 5
Time Complexity: O(logn) due to traversal in toggle() function
Space Complexity: O(1)
Approach 3: Bit Mask
Logically XNOR is inverse of XOR, but upon performing complement of XOR, leading zeroes are also inverted. It is avoided using a bit mask that removes all the unnecessary leading bits.
Sample Example 1
Input: x = 12, y = 5
Output: 6
Explanation
(12)10 = (1100)2 (5)10 = (101)2 1100 ^ 101 = 1001 Inverse of 1001 = 0110
Sample Example 2
Input: x = 12, y = 31
Output: 12
Explanation
(12)10 = (1100)2 (31)10 = (11111)2 1100 ^ 11111 = 10011 Inverse of 10011 = 01100
Psuedocode
Procedure xnor (x, y) bit_count = log2 (maximum of a and y) mask = (1 << bit_count) - 1 ans = inverse(x xor y) and mask end procedure
Example: C++ Implementation
In the following program, bit mask is used to obtain only the required bits from inverse of x xor y.
#include <bits/stdc++.h> using namespace std; // Function to find the XNOR of two numbers int xnor(int x, int y){ // Maximum number of bits used in both the numbers int bit_count = log2(max(x, y)); int mask = (1 << bit_count) - 1; // Inverse of XOR operation int inv_xor = ~(x ^ y); // GEtting the required bits and removing the inversion of leading zeroes. return inv_xor & mask; } int main(){ int x = 10, y = 10; cout << "XNOR of " << x << " and " << y << " = " << xnor(x, y); return 0; }
Output
XNOR of 10 and 10 = 7
Conclusion
In conclusion, XNOR of two numbers can be found using various approaches and time complexities ranging from O(logn) which brute force to O(1) which is most oprimized. Applying bit operations is cheaper thus the brute force complexity is logarithmic.