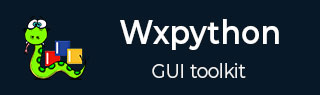
- wxPython Tutorial
- wxPython - Home
- wxPython - Introduction
- wxPython - Environment
- wxPython - Hello World
- wxPython - GUI Builder Tools
- wxPython - Major Classes
- wxPython - Event Handling
- wxPython - Layout Management
- wxPython - Buttons
- wxPython - Dockable Windows
- Multiple Document Interface
- wxPython - Drawing API
- wxPython - Drag and Drop
- wxPython Resources
- wxPython - Quick Guide
- wxPython - Useful Resources
- wxPython - Discussion
wxPython - ComboBox & Choice Class
A wx.ComboBox object presents a list of items to select from. It can be configured to be a dropdown list or with permanent display.
The selected item from the list is displayed in a text field, which by default is editable, but can be set to be read-only in the presence of wx.CB_READONLY style parameter.
wxPython API contains a wx.Choice class, whose object is also a dropdown list, which is permanently read-only.
The parameters used by wx.ComboBox class constructor are −
Wx.ComboBox(parent, id, value, pos, size, choices[], style)
The value parameter is the text to be displayed in the text box of combobox. It is populated from the items in choices[] collection.
The following style parameters are defined for wx.ComboBox −
S.N. | Parameters & Description |
---|---|
1 | wx.CB_SIMPLE Combobox with permanently displayed list |
2 | wx.CB_DROPDOWN Combobox with dropdown list |
3 | wx.CB_READONLY Chosen item is not editable |
4 | wx.CB_SORT List is displayed in alphabetical order |
The following table shows commonly used methods of wx.ComboBox class −
S.N. | methods & Description |
---|---|
1 | GetCurrentSelection () Returns the item being selected |
2 | SetSelection() Sets the item at the given index as selected |
3 | GetString() Returns the string associated with the item at the given index |
4 | SetString() Changes the text of item at the given index |
5 | SetValue() Sets a string as the text displayed in the edit field of combobox |
6 | GetValue() Returns the contents of the text field of combobox |
7 | FindString() Searches for the given string in the list |
8 | GetStringSelection() Gets the text of the currently selected item |
Event binders for events generated by this class are as follows −
S.N. | Events & Description |
---|---|
1 | wx. COMBOBOX When item from the list is selected |
2 | wx. EVT_TEXT When combobox text changes |
3 | wx. EVT_COMBOBOX_DROPDOWN When list drops down |
4 | wx. EVT_COMBOBOX_CLOSEUP When list folds up |
wx.Choice class constructor prototype is as follows −
wx.Choice(parent, id, pos, size, n, choices[], style)
Parameter ānā stands for number of strings with which the choice list is to be initialized. Like comboBox, the list is populated with items in choices[] collection.
For Choice class, only one style parameter is defined. It is wx.CB_SORT. Only one event binder processes the event emitted by this class. It is wx.EVT_CHOICE.
Example
This example displays the features of wx.ComboBox and wx.Choice. Both objects are put in a vertical box sizer. The lists are populated with items in languages[] List object.
languages = ['C', 'C++', 'Python', 'Java', 'Perl'] self.combo = wx.ComboBox(panel,choices = languages) self.choice = wx.Choice(panel,choices = languages)
Event binders EVT_COMBOBOX and EVT_CHOICE process corresponding events on them.
self.combo.Bind(wx.EVT_COMBOBOX, self.OnCombo) self.choice.Bind(wx.EVT_CHOICE, self.OnChoice)
The following handler functions display the selected item from the list on the label.
def OnCombo(self, event): self.label.SetLabel("selected "+ self.combo.GetValue() +" from Combobox") def OnChoice(self,event): self.label.SetLabel("selected "+ self.choice. GetString( self.choice.GetSelection() ) +" from Choice")
The complete code listing is as follows −
import wx class Mywin(wx.Frame): def __init__(self, parent, title): super(Mywin, self).__init__(parent, title = title,size = (300,200)) panel = wx.Panel(self) box = wx.BoxSizer(wx.VERTICAL) self.label = wx.StaticText(panel,label = "Your choice:" ,style = wx.ALIGN_CENTRE) box.Add(self.label, 0 , wx.EXPAND |wx.ALIGN_CENTER_HORIZONTAL |wx.ALL, 20) cblbl = wx.StaticText(panel,label = "Combo box",style = wx.ALIGN_CENTRE) box.Add(cblbl,0,wx.EXPAND|wx.ALIGN_CENTER_HORIZONTAL|wx.ALL,5) languages = ['C', 'C++', 'Python', 'Java', 'Perl'] self.combo = wx.ComboBox(panel,choices = languages) box.Add(self.combo,1,wx.EXPAND|wx.ALIGN_CENTER_HORIZONTAL|wx.ALL,5) chlbl = wx.StaticText(panel,label = "Choice control",style = wx.ALIGN_CENTRE) box.Add(chlbl,0,wx.EXPAND|wx.ALIGN_CENTER_HORIZONTAL|wx.ALL,5) self.choice = wx.Choice(panel,choices = languages) box.Add(self.choice,1,wx.EXPAND|wx.ALIGN_CENTER_HORIZONTAL|wx.ALL,5) box.AddStretchSpacer() self.combo.Bind(wx.EVT_COMBOBOX, self.OnCombo) self.choice.Bind(wx.EVT_CHOICE, self.OnChoice) panel.SetSizer(box) self.Centre() self.Show() def OnCombo(self, event): self.label.SetLabel("You selected"+self.combo.GetValue()+" from Combobox") def OnChoice(self,event): self.label.SetLabel("You selected "+ self.choice.GetString (self.choice.GetSelection())+" from Choice") app = wx.App() Mywin(None, 'ComboBox and Choice demo') app.MainLoop()
The above code produces the following output −
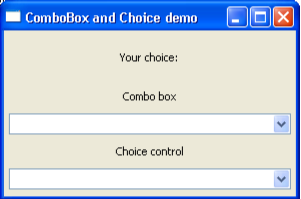