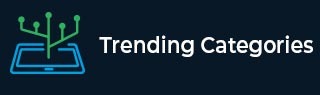
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Writing C/C++ code efficiently in Competitive programming
In competitive programming, the most important thing is an effective code. Optimized and faster code is important and can make a difference in the ranks of the programmer.
To write an effective c/c++ code in competitive programming, here are some effective tools for writing c/c++ code efficiently,
First, let’s recall some basic terms,
Template is writing code that does not depend on a particular type.
Macro is a named code fragment.
Vectors are like automatically resizable dynamic arrays that update size with insertion and deletion of the element.
Now, let’s see some basic updates in code that can make in increasing efficiency of code,
Using faster input/output method is c/c++ − scanf/printf are faster input-output methods.
Assigning variables with maximum and minimum value − using min-max function decreases efforts.
Creating Range based loops − For effective coding, iterating in a loop using ranged loops over array and vectors.
Example
#include<iostream> using namespace std; int main() { cout<<"Iterating over array using ranged array: "; int array[]= {6, 10, 31, 17, 50}; for (const auto &value: array) cout<<value<<" "; return 0; }
Output
Iterating over array using ranged array: 6 10 31 17 50
- Using proper header files − generally in c++ programming has a lot of different libraries that are used to include different functionalities to the program. The header file : #include<bits/stdc++.h> has almost all files that are need in competitive programming. But while programming makes sure you need some of these functions before including this library. Otherwise, it may take up computing space.
Use containers to reduce space − containers like vectors, maps, lists, etc that are used for solving many problems in competitive programming. The predefined functions on these containers reduce the size of code and make it more effective.
Use auto to declare data types, because their types are defined at compile time.