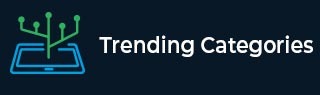
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Write a program in Python to print the elements in a series between a specific range
Input − Assume, you have a series,
0 12 1 13 2 15 3 20 4 19 5 18 6 11
Output − The result for the elements between 10 to 15 as,
0 12 1 13 2 15 6 11
Solution 1
Define a Series
Create an empty list.
Create for loop to access all the elements one by one and set if condition to compare the value from above or equal to 10 and below or equal to 15. Append matched values to an empty list as follows −
for i in range(len(data)): if(data[i]>=10 and data[i]<=15): ls.append(data[i])
Finally, check the list of values to the series using isin().
Example
Let us see the following implementation to get a better understanding.
import pandas as pd l = [12,13,15,20,19,18,11] data = pd.Series(l) print(data[data.between(10,15)])
Output
0 12 1 13 2 15 6 11
Solution 2
Example
import pandas as pd l = [12,13,15,20,19,18,11] data = pd.Series(l) ls = [] for i in range(len(data)): if(data[i]>=10 and data[i]<=15): ls.append(data[i]) print(data[data.isin(ls)])
Output
0 12 1 13 2 15 6 11
Advertisements