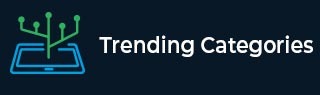
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Write a Golang program to swap two numbers without using a third variable
Approach to solve this problem
- Step 1: Define a function that accepts two numbers, type is int.
- Step 2: Find b = a + b;
- Step 3: Then a = b – a and b = b – a
Program
package main import "fmt" func swap(a, b int){ fmt.Printf("Before swapping, numbers are %d and %d\n", a, b) b = a + b a = b - a b = b - a fmt.Printf("After swapping, numbers are %d and %d\n", a, b) } func main(){ swap(23, 45) swap(56, 100) }
Output
Before swapping, numbers are 23 and 45 After swapping, numbers are 45 and 23 Before swapping, numbers are 56 and 100 After swapping, numbers are 100 and 56
Advertisements