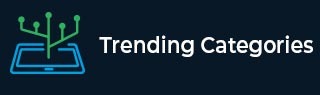
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Write a Golang program to find the sum of digits for a given number
Examples
- num = 125 => 1 + 2 + 5 = 8
- num = 101 => 1 + 0 + 1 = 2
- num = 151 => 1 + 5 + 1 = 7
Approach to solve this problem
Step 1: Define a function that accepts numbers(num); type is int.
Step 2: Start a true loop until num becomes 0 and define res:=0.
Step 3: Find modulo and add to res.
Step 4: Divide num by 10.
Step 5: Return res.
Program
package main import "fmt" func findDigitSum(num int) int { res := 0 for num>0 { res += num % 10 num /= 10 } return res } func main(){ fmt.Println(findDigitSum(168)) fmt.Println(findDigitSum(576)) fmt.Println(findDigitSum(12345)) }
Output
15 18 15
Advertisements