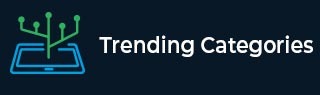
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Write a Code to Sort an Array in Numpy by the (N-1)Th Column
In this example, we will see how to sort an array in Numpy by the (N-1)th column.
Sort an array by (n-1) th column using argsort()
Example
Let us see the first example to sort an array by (n-1)th column −
import numpy as np # Creat a Numpy Array a = np.array([[9, 2, 3], [4, 5, 6], [7, 0, 5]]) print("Array = \n",a) # The value of n n = 3 # Sort by n-1 column print("\nSort by n-1 th column = \n",a[a[:, (n-1)].argsort()])
Output
Array = [[9 2 3] [4 5 6] [7 0 5]] Sort by n-1 th column = [[9 2 3] [7 0 5] [4 5 6]]
Sort an array by (n-1) th column using sorted() and lambda
Example
In this example, we will sort using lambda −
import numpy as np # Create a Numpy Array arr = np.array([[5, 7, 7], [2, 3, 9], [7, 6, 6]]) print("Array = \n",arr) # The value of n n = 3 # Sort by n-1 column using lambda arr = sorted(arr, key=lambda arr_entry: arr_entry[n-1]) print("\nSort by n-1 th column = \n",arr)
Output
Array = [[5 7 7] [2 3 9] [7 6 6]] Sort by n-1 th column = [array([7, 6, 6]), array([5, 7, 7]), array([2, 3, 9])]
Sort an array by (n-1) th column using mergesort
Example
In this example, we will sort using the mergesort() method in Python −
import numpy as np # Create a Numpy Array arr = np.array([[5, 7, 7], [2, 3, 9], [7, 6, 6]]) print("Array = \n",arr) # The value of n n = 3 # Sort by n-1 column using mergesort arr = arr[arr[:,(n-1)].argsort(kind='mergesort')] print("\nSort by n-1 th column = \n",arr)
Output
Array = [[5 7 7] [2 3 9] [7 6 6]] Sort by n-1 th column = [[7 6 6] [5 7 7] [2 3 9]]
Advertisements