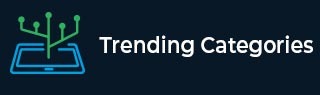
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Write a C program to display the size and offset of structure members
Problem
Write a C program to define the structure and display the size and offsets of member variables
Structure − It is a collection of different datatype variables, grouped together under a single name.
General form of structure declaration
datatype member1; struct tagname{ datatype member2; datatype member n; };
Here, struct - keyword
tagname - specifies name of structure
member1, member2 - specifies the data items that make up structure.
Example
struct book{ int pages; char author [30]; float price; };
Structure variables
There are three ways of declaring structure variables −
Method 1
struct book{ int pages; char author[30]; float price; }b;
Method 2
struct{ int pages; char author[30]; float price; }b;
Method 3
struct book{ int pages; char author[30]; float price; }; struct book b;
Initialization and accessing of structures
The link between a member and a structure variable is established using the member operator (or) dot operator.
Initialization can be done in the following ways −
Method 1
struct book{ int pages; char author[30]; float price; } b = {100, "balu", 325.75};
Method 2
struct book{ int pages; char author[30]; float price; }; struct book b = {100, "balu", 325.75};
Method 3 (using member operator)
struct book{ int pages; char author[30]; float price; } ; struct book b; b. pages = 100; strcpy (b.author, "balu"); b.price = 325.75;
Method 4 (using scanf function)
struct book{ int pages; char author[30]; float price; } ; struct book b; scanf ("%d", &b.pages); scanf ("%s", b.author); scanf ("%f", &b. price);
Declare the structure with data members and try to print their offset values as well as size of the structure.
Program
#include<stdio.h> #include<stddef.h> struct tutorial{ int a; int b; char c[4]; float d; double e; }; int main(){ struct tutorial t1; printf("the size 'a' is :%d
",sizeof(t1.a)); printf("the size 'b' is :%d
",sizeof(t1.b)); printf("the size 'c' is :%d
",sizeof(t1.c)); printf("the size 'd' is :%d
",sizeof(t1.d)); printf("the size 'e' is :%d
",sizeof(t1.e)); printf("the offset 'a' is :%d
",offsetof(struct tutorial,a)); printf("the offset 'b' is :%d
",offsetof(struct tutorial,b)); printf("the offset 'c' is :%d
",offsetof(struct tutorial,c)); printf("the offset 'd' is :%d
",offsetof(struct tutorial,d)); printf("the offset 'e' is :%d
",offsetof(struct tutorial,e)); printf("size of the structure tutorial is :%d",sizeof(t1)); return 0; }
Output
the size 'a' is :4 the size 'b' is :4 the size 'c' is :4 the size 'd' is :4 the size 'e' is :8 the offset 'a' is :0 the offset 'b' is :4 the offset 'c' is :8 the offset 'd' is :12 the offset 'e' is :16 size of the structure tutorial is :24
Advertisements