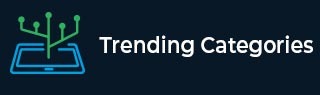
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
What is the use of parsing dates in JavaScript?
The date. parse() method parsing a string representation of a date, and returns the number of milliseconds.
parse() parse a date string and returns the time difference between midnight 1, 1970 to provided date.
The date. parse() method is used to know the exact number of milliseconds that have passed from midnight, January 1, 1970, till the date we provide.
Syntax
Following is the syntax of the parse() function −
Date.parse(dateString)
This method accepts only one parameter and holds the date as a string. It returns an integer value that represents the number of milliseconds between January 1, 1970, and the date provided. The machine can’t recognize the string or the input string is invalid. It will return not a 'NaN' instead of an integer.
Example: 1
If the input of the date is not correct, then it will return 'NaN' i.e, not a number.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8" /> <meta http-equiv="X-UA-Compatible" content="IE=edge" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <title>parsing date</title> </head> <body> <script> var date = "February 32, 2018 12:30 PM"; var msec = Date.parse(date); document.write(msec); </script> </body> </html>
Example 2
In the following example, we are calculating the number of years b/w January 1, 1970 and provided date.
<!DOCTYPE html> <html> <head> </head> <body> <p>Calculate the number of years between 19th July 2022 and January 1, 1970:</p> <p id="demo"></p> <script> const minute = 1000 * 60; const hour = minute * 60; const day = hour * 24; const year = day * 365; const date = Date.parse("July 19, 2022"); let years = Math.round(date / year); document.getElementById("demo").innerHTML = years; </script> </body> </html>
Example 3
In the following example, we are calculating the number of milliseconds when we were parsing the date.
<!DOCTYPE html> <!DOCTYPE html> <html lang="en"> <head> <title>parsing date</title> </head> <body> <script> var date = "July 19, 2022"; var msec = Date.parse(date); document.write(msec); </script> </body> </html>
Example 4
In the following example, we are getting the present date with the help of a new Date() method and after that, we were parsing the date and finding the millisecond.
<!DOCTYPE html> <!DOCTYPE html> <html lang="en"> <head> <title>parsing date</title> </head> <body> <script> var date = new Date(); document.write(" The present date is: " + date + "</br>"); var msec = Date.parse(date); document.write(msec); </script> </body> </html>