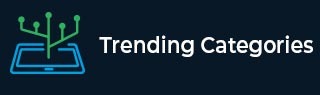
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
What is the use of Object.is() method in JavaScript?
Object.is()
Object.is() is used to check whether two values are same or not.
Two values are same when they have the following criteria.
- Either both the values are undefined or null .
- Either both are true or false.
- Both strings should be of same length, same characters and in same order.
- The polarities of both the values should be equal.
- Both the values can be NaN and should be equal.
syntax
Object.is(val1, val2);
It accepts two parameters and scrutinize whether they are equal or not. If equal gives out true as output else false as output.
There is a small difference between Object.is() and "==" that is when comparing +0 and -0, the former results false whereas the latter results true. From this we can conclude that the method Object.is() scrutinizes even polarity.
Example
<html> <body> <script> // comparing strings with same characters and same order var val = Object.is("tutorialspoint", "tutorialspoint") document.write(val); document.write("</br>"); // comparing polarity var pol = Object.is(-0, +0) document.write(pol); document.write("</br>"); //comparing unequal strings var uneq = Object.is("tutorialspoint!", "tutorialspoint") document.write(uneq); document.write("</br>"); // comparing objects var obj = Object.is( {object : 1}, {object : 2}) document.write(obj); </script> </body> </html>
Output
true false false false
Advertisements