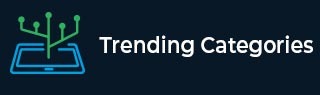
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
What is the syntax for passing Scanner object as a parameter in a method using java?
Until Java 1.5 to read data from the user programmers used to depend on the character stream classes and byte stream classes.
From Java 1.5 Scanner class was introduced. This class accepts a File, InputStream, Path and, String objects, reads all the primitive data types and Strings (from the given source) token by token using regular expressions.
By default, whitespace is considered as the delimiter (to break the data into tokens).
To read various datatypes from the source using the nextXXX() methods provided by this class namely, nextInt(), nextShort(), nextFloat(), nextLong(), nextBigDecimal(), nextBigInteger(), nextLong(), nextShort(), nextDouble(), nextByte(), nextFloat(), next().
Passing Scanner object as a parameter
You can pass Scanner object to a method as a parameter.
Example
The following Java program demonstrates how to pass a Scanner object to a method. This object reads the contents of a file.
import java.io.FileInputStream; import java.io.IOException; import java.io.InputStream; import java.util.Scanner; public class ScannerExample { public String sampleMethod(Scanner sc){ StringBuffer sb = new StringBuffer(); while(sc.hasNext()) { sb.append(sc.nextLine()); } return sb.toString(); } public static void main(String args[]) throws IOException { //Instantiating the inputStream class InputStream stream = new FileInputStream("D:\sample.txt"); //Instantiating the Scanner class Scanner sc = new Scanner(stream); ScannerExample obj = new ScannerExample(); //Calling the method String result = obj.sampleMethod(sc); System.out.println("Contents of the file:"); System.out.println(result); } }
Output
Contents of the file: Tutorials Point originated from the idea that there exists a class of readers who respond better to on-line content and prefer to learn new skills at their own pace from the comforts of their drawing rooms.
Example
In the following example, we creating a Scanner object which has Standard input (System.in) as source and passing it as a parameter to a method.
import java.io.IOException; import java.util.Scanner; public class ScannerExample { public void sampleMethod(Scanner sc){ StringBuffer sb = new StringBuffer(); System.out.println("Enter your name: "); String name = sc.next(); System.out.println("Enter your age: "); String age = sc.next(); System.out.println("Hello "+name+" You are "+age+" years old"); } public static void main(String args[]) throws IOException { //Instantiating the Scanner class Scanner sc = new Scanner(System.in); ScannerExample obj = new ScannerExample(); //Calling the method obj.sampleMethod(sc); } }
Output
Enter your name: Krishna Enter your age: 25 Hello Krishna You are 25 years old