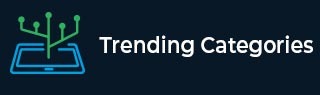
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
What is the purpose of using Optional.ifPresentOrElse() method in Java 9?
The improvement of ifPresentOrElse() method in Optional class is that accepts two parameters, Consumer and Runnable. The purpose of of using ifPresentOrElse() method is that if an Optional contains a value, the function action is called on the contained value, i.e. action.accept (value), which is consistent with ifPresent() method. The difference from the ifPresent() method is that ifPresentOrElse() has a second parameter, emptyAction. If Optional contains no value, then ifPresentOrElse() method calls emptyAction, i.e. emptyAction.run().
Syntax
public void ifPresentOrElse(Consumer<? super T> action, Runnable emptyAction)
Example
import java.util.Optional; public class IfPresentOrElseMethodTest { public static void main(String args[]) { Optional<Integer> optional = Optional.of(1); optional.ifPresentOrElse(x -> System.out.println("Value: " + x), () -> System.out.println("Not Present")); optional = Optional.empty(); optional.ifPresentOrElse(x -> System.out.println("Value: " + x), () -> System.out.println("Not Present")); } }
Output
Value: 1 Not Present
Advertisements