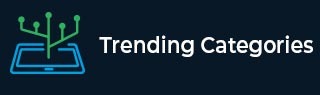
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
What is the preferred method to check for an empty array in NumPy?
In this article, we will show you the preferred methods to check for an empty array in Numpy.
NumPy is a Python library designed to work efficiently with arrays in Python. It is fast, simple to learn, and efficient in storage. It also improves the way data is handled for the process. In NumPy, we may generate an n-dimensional array. To use NumPy, we simply import it into our program, and then we can easily use NumPy's functionality in our code.
NumPy is a popular Python package for scientific and statistical analysis. NumPy arrays are grids of values from the same data type.
Using numpy.any() method
The numpy.any() function determines if any array element along a given axis evaluates to True.
Syntax
numpy.any(a, axis = None, out = None, keepdims = <no value>)
Parameters
a − Input array whose elements must be checked.
axis − It is an axis along which array elements are evaluated.
out − Output array with the same dimensions as the input array.
keepdims − If this is set to True, the reduced axes are kept in the result.
Return Value − returns a new Boolean array depending on the ‘out’ argument
Algorithm (Steps)
Following are the Algorithm/steps to be followed to perform the desired task −
Use the import keyword, to import the numpy module with an alias name(np).
Use array() function(returns an ndarray. The ndarray is an array object that satisfies the given requirements) of the numPy module to create a numpy array.
Use the numpy.any() function(The numpy.any() function determines if any array members along the specified axis are True) to check whether the input array is empty or Not i.e returns True.
If the any() function returns False then the given array is empty otherwise it is not empty.
Example
The following program returns whether the given NumPy array is empty or not using the numpy.any() function −
# importing NumPy module with an alias name import numpy as np # creating a NumPy array inputArray = np.array([]) # Checking whether the input array is empty or not using any() function # Here it returns false if the array is empty else it returns true temp_flag = np.any(inputArray) # checking whether the temp_flag is false (Numpy array is empty) if temp_flag == False: # printing empty array, if the condition is true print('Empty NumPy Input Array') else: # else printing NOT Empty print('Input NumPy Array is NOT Empty')
Output
On executing, the above program will generate the following output −
Empty NumPy Input Array
Using numpy.size() method
To count the number of elements along a given axis, we use Python's numpy.size() method.
Syntax
numpy.size(a, axis=None)
Parameters
a − Input array.
axis − It is an axis along which array elements are counted.
Return Value − returns the number of elements along the specified axis.
Example
The following program returns whether the given NumPy array is empty or not using the numpy.size() function −
# importing numpy module with an alias name import numpy as np # creating a numpy array inputArray = np.array([]) # Getting the size of inputArray arraySize = np.size(inputArray) # Checking whether the array size is 0(empty array) or not(array containing elements) if arraySize==0: # printing empty array, if the condition is true print('Empty NumPy Input Array') else: # else printing NOT Empty print('Input NumPy Array is NOT Empty')
Output
On executing, the above program will generate the following output −
Empty NumPy Input Array
By Converting Numpy array into the list
In this method, we first use the tolist() method to convert the array into a list. The length of the list was then checked using the len() method to see if the array was empty.
Example
The following program returns whether the given NumPy array is empty or not using the len() function −
# importing numpy module with an alias name import numpy as np # creating an array inputArray = np.array([]) # converting NumPy array to list and checking # whether the length of the list is equal to 0 if len(inputArray.tolist()) == 0: # Print Empty if condition is true print("Empty input array") else: # else printing not Empty array print('Input array is NOT empty')
Output
On executing, the above program will generate the following output −
Empty input array
Using size attribute method
size attribute −This attribute computes the total number of elements in the NumPy array.
Example
The following program returns whether the given NumPy array is empty or not using the size attribute −
import numpy as np # creating a NumPy array inputArray = np.array([]) # checking whether the size of the array is equal to 0 if inputArray.size == 0: # prining empty array if condition is true print("Empty input array") else: # else printing not Empty array print('Input array is NOT empty')
Output
On executing, the above program will generate the following output −
Empty input array
In this method, we used the inputArray.size attribute to determine whether or not the array is empty. This operator returns the array size, which in this example is 0, resulting in the expected result.
Using shape attribute
This is a numpy array attribute that gives a tuple containing the array's shape. This can be used to see if the array is empty.
Example
The following program returns whether the given NumPy array is empty or not using the shape attribute −
import numpy as np # creating a numpy array inputArray = np.array([]) # checking whether the shape of the array is equal to 0 (Empty array condition) if inputArray.shape[0] == 0: # prining empty array if condition is true print("Empty input array") else: # else printing not Empty array print('Input array is NOT empty')
Output
On executing, the above program will generate the following output −
Empty input array
Conclusion
This article taught us 5 distinct types of preferred methods for determining whether a given NumPy array is empty or not.