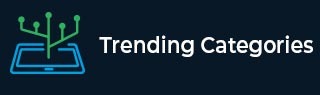
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
What is the difference between undefined and not defined in JavaScript?
In JavaScript, there are two main ways that a variable can be "undefined". The first is when you declare a variable without giving it a value. The second is when you try to access a variable that does not exist.
Undefined in JavaScript
When a variable is declared without a value, it is automatically given the value of "undefined". This can happen if you forget to assign a value to a variable, or if you intentionally do not assign a value (For example, if you are waiting for user input).
If you try to access a variable that does not exist, you will also get the value of "undefined". This can happen if you type the name of a variable, or if you are trying to access a variable that is out of scope.
Example 1
Below is the example with an explanation.
<html> <head> <title>Examples</title> </head> <body> <div id="result"></div> <script> var foo; document.getElementById("result").innerHTML = foo; </script> </body> </html>
In the above example, the variable "foo" is declared but not given a value. Therefore, when we try to access it, the value of "undefined" is returned.
Example 2
<html> <head> <title>Examples</title> </head> <body> <div id="result"></div> <script> var myObj = {}; document.getElementById("result").innerHTML = myObj.foo; </script> </body> </html>
In the above example, we are trying to access the property "foo" of the object "myObj". However, since this property does not exist, the value of "undefined" is returned.
Not defined in JavaScript
The value of "not defined" is similar to "undefined", in that it indicates that a variable does not exist. However, there is a subtle difference between the two.
"not defined" is usually caused by a typo, or by trying to access a variable that is out of scope. "undefined" is usually caused by forgetting to assign a value to a variable.
Example
Below is the example with an explanation.
<html> <head> <title>Examples</title> </head> <body> <div id="result"></div> <script> var myObj = {}; try { document.getElementById("result").innerHTML = myOb.foo; } catch(err) { document.getElementById("result").innerHTML = err; } </script> </body> </html>
In the above example, we are trying to access the property "foo" of the object "myOb". However, since this object does not exist, the ReferenceError of "myOb is not defined" is returned.
The difference between undefined and not defined
The main difference between "undefined" and "not defined" is that "undefined" is a value that can be assigned to a variable, while "not defined" indicates that a variable does not exist.
Another difference is that "undefined" is usually caused by forgetting to assign a value to a variable, while "not defined" is usually caused by a typo or by trying to access a variable that is out of scope.
Conclusion
In conclusion, "undefined" and "not defined" are two different values in JavaScript. "undefined" indicates that a variable has been declared but not given a value, while "not defined" indicates that a variable does not exist.