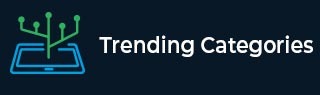
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
What is the default value of a local variable in Java?
The local variables can be declared in methods, code blocks, constructors, etc in Java. When the program control enters the methods, code blocks, constructors, etc. then the local variables are created and when the program control leaves the methods, code blocks, constructors, etc. then the local variables are destroyed. The local variables do not have any default values in Java. This means that they can be declared and assigned a value before the variables are used for the first time, otherwise, the compiler throws an error.
Example
public class LocalVariableTest { public void print() { int num; System.out.println("The number is : " + num); } public static void main(String args[]) { LocalVariableTest obj = new LocalVariableTest(); obj.print(); } }
In the above program, a local variable num can't be initialized with a value, so an error will be generated like “variable num might not have been initialized”.
Output
LocalVariableTest.java:4: error: variable num might not have been initialized System.out.println("The number is : " + num); ^ 1 error
Example
public class LocalVariableTest { public void print() { int num = 100; System.out.println("The number is : " + num); } public static void main(String args[]) { LocalVariableTest obj = new LocalVariableTest(); obj.print(); } }
In the above program, a local variable "num" can be initialized with a value of '100'
Output
The number is : 100