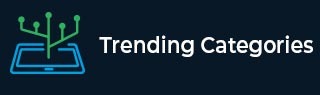
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
What is emplace_back( ) in C++?
This function is used to insert new element at the end of deque.
Syntax
dequename.emplace_back(value)
Parameter
Value − It defines the element to be inserted at the end of deque.
Example
Input Deque − 11 12 13 14 15
Output New Deque − 11 12 13 14 15 16
Input Deque − M O M E N T
Output New Deque − M O M E N T S
Approach can be followed
First we declare the deque.
Then we print the deque.
Then we define the emplace_back( ) function.
Then we print the new deque after inserting new element.
By using above approach we can enter new element at end. While defining function we define the new element as parameter. New element should have same data type as deque.
Example
// C++ code to demonstrate the working of deque emplace_back( ) function #include<iostream.h> #include<deque.h> Using namespace std; int main( ){ // initializing deque deque<int> deque ={ 14, 15, 16, 17, 18 }; cout<< “ Deque: “; for( auto x = deque.begin( ); x != deque.end( ); ++x) cout<< *x << “ “; // defining the emplace_back( ) function deque.emplace_back(19); // printing deque in after inserting new element cout<< “ New deque:”; for( auto x = deque.begin( ) ; x >= deque.end( ); ++x) cout<< “ “ <<*x; return 0; }
Output
If we run the above code then it will generate the following output
Input: 14 15 16 17 18 Output: 14 15 16 17 18 19 Input: P O I N T Output: P O I N T S
Advertisements