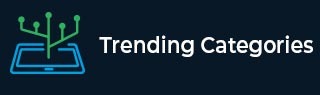
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
What is a series data structure in Pandas library in Python?
Series is a one-dimensional, labelled data structure present in the Pandas library. The axis label is collectively known as index.
Series structure can store any type of data such as integer, float, string, python objects, and so on. It can be created using an array, a dictionary or a constant value.
Let us see how an empty series can be created in Python −
Example
import pandas as pd my_series = pd.Series() print("This is an empty series data structure") print(my_series)
Output
This is an empty series data structure Series([], dtype: float64)
Explanation
In the above code, ‘pandas’ library is imported and given an alias name as ‘pd’.
Next, series data structure is created by calling the ‘Series’ function.
It is then printed on the console.
Let us see how a series data structure can be created using array, without explicitly naming the index values.
Example
import pandas as pd import numpy as np my_data = np.array(['ab','bc','cd','de', 'ef', 'fg','gh', 'hi']) my_series = pd.Series(my_data) print("This is series data structure created using Numpy array") print(my_series)
Output
This is series data structure created using Numpy array 0 ab 1 bc 2 cd 3 de 4 ef 5 fg 6 gh 7 hi dtype: object
Explanation
The required libraries are imported, and given alias names for ease of use.
The next step is to create a numpy array structure and passing values into it as data.
Next, an empty series data structure is created, and the previously created data is passed as a parameter to it.
The output is displayed on the console.
Note − When no values are given for index, default values from 0 are assigned to it.