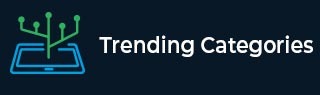
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
What is a Python module? How is it different from libraries?
It can be difficult for beginners to grasp the concept of Python modules and libraries. You can tell from the zoom-out content that each of them is a collection of codes. But there is a significant difference between them.
In this article, we will show you the major difference between python modules and libraries.
Python Modules and Libraries
Real-world programs are complicated. Even simple software contains thousands of lines of code. Because of this, writing code in continuous flow is difficult for programmers and developers to grasp. Developers utilize modular programming to facilitate learning and make it logically separated. It is a method of breaking down huge coding tasks into shorter, more logical, and more adaptable subtasks.
Python's ease of use is one of its primary goals. Python has so many modules and libraries because of this.
Modules in Python
Modules are collections of related code that are packaged together in a Python program. Within a module, programmers can define functions, classes, or variables. It’s also great to accommodate runnable codes within modules. They are, in other words, Python files that contain valid Python definitions and statements. When these files are created, the suffix.py is appended to them. By grouping related code into modules, the code becomes easier to understand and implement. It also organizes the code in a logical manner.
Modules are divided into two types.
- Predefined Modules(Built-in Modules)
- User-defined Modules
- External Modules
Predefined Modules
These are also referred to as built-in modules. Python has a huge number of built-in modules. Programmers can utilize these modules in Python applications by invoking their names along with the keyword 'import'. Import math, for example. The majority of the built-in Python modules are written in C and then combined with the Python interpreter to allow the interpreter to work alongside them.
Math, datetime, statistics, random, os, sys, and other popular built-in Python modules.
Example
# importing sqrt, factorial functions from math module from math import sqrt, factorial # printing the square root of 16 using sqrt() function print('The square root of 16 = ',sqrt(16)) # printing the factorial of 5 using factorial() function print('The factorial of 5 = ',factorial(5))
Output
On executing, the above program will generate the following output −
('The square root of 16 = ', 4.0) ('The factorial of 5 = ', 120)
User-defined Modules
Another advantage that Python gives its programmers is the ability to create user-defined modules. Python enables programmers to customize their own operations and gain control over them. Programmers can design their own collection of functions, variables, and classes within a user-defined module.
The technique for importing user-defined modules is the same as for predefined modules.
Example
sampleModule.py
def sampleFunction(givenValue): print("The values passed to the function is:", givenValue)
compiler code
import sampleModule sampleModule.sampleFunction(10)
Output
On executing, the above program will generate the following output −
First, we made a module called sampleModule.py Within the module, we defined a function called sampleFunction, which takes a value as an argument and prints it.
After that, we used a compiler to import the previously created module sampleModule. After we've imported the module, we can use the (.) operator to access all of its functions.
So we called the sampleFunction of the sampleModule by passing it some random value as an argument, and the function then prints the value of the argument.
External Modules
External modules must be downloaded from outside. They don't exist yet like the built-in ones. Installing them is a very simple task and can be done using the "pip install module_name" command in the compiler terminal. With so many modules available, familiarity with all seems to go a long way even for the best programmers. So you can search for modules to find and use them as needed. You don't have to remember everything, just search the web when you need it.
Example
Emoji module
Emoji have become a way to express and enhance the simple, boring text. The same gem can now be used in Python programs as well. Yes, it is! Now you have the ultimate way to use emojis in your code. To do this, you need to install the Emoji module.
Installation
pip install emoji
The following program prints the emoji using the emoji module −
# Importing emojize function from emoji module from emoji import emojize # Passing name of emoji as an argument to the emojize() function print(emojize(":thumbs_up:"))
Output
On executing, the above program will generate the following output −
Libraries
A library is an umbrella term that comprises a reusable set of Python code/instructions.
A Python library is typically a collection of similar modules grouped together under a single name. Developers commonly utilize it to share reusable code with the community. This eliminates the need to write Python code from scratch.
Developers and community researchers can construct their own set of useful functions in the same domain. When programmers and developers install the Python interpreter on their machines, standard libraries are included. Python libraries include matplotlib, Pygame, Pytorch, Requests, Beautifulsoup, and others.
Example
# impoting matplotlib library with an alias name import matplotlib.pyplot as matplot # input first list inputList_1 = [1, 4, 6, 8] # input first list inputList_2 = [2, 6, 3, 9] # plotting the inputList_1, inputList_2 values by taking inputList_1 on x-axis # and inputList_2 on y-axis. matplot.plot(inputList_1, inputList_2) # giving the label/name for x-axis matplot.xlabel('X-axis') # giving the label/name for y-axis matplot.ylabel('Y-axis') # giving the title of the plot matplot.title('Plot for Data Visualization') # displaying the graph matplot.show()
Output
On executing, the above program will generate the following output −
Difference Between Modules and Libararies
The following table shows the major differences between modules and libraries in python −
Modules | Libraries |
---|---|
A module is a set of code or functions with the.py extension. |
A library is a collection of related modules or packages. |
They are used by both programmers and developers. |
Libraries are used by community members, developers and researchers. |
The use of modules makes it easier to read the code. |
Libraries provide no contribution for easy readability. |
Modules are logical groups of functionality that programmers can import in order to reuse their code or set of statements. |
Libraries enable users of programming languages, developers, and other researchers to reuse collections of logically related code. |
When a Python programmer imports a module, the interpreter searches various locations for the module's definition or body. |
Before we can use the libraries' modules or packages, we must first install them in our Python project. We generally use the pip install command. |
Modules are generally written in Python with valid statements or codes. |
Libraries, especially standard libraries, are usually developed in C or Python. |
The basic goal of creating a module is to prevent DRY i.e, Don't Repeat Yourself. |
Libraries do not have such a goal. |
To return a sorted list of strings containing the function names specified within a module, we can use Python's built-in dir() function. |
There is no explicit function that returns the number of modules in a library. Even so, programmers can utilize the help() function to get some information. |
Popular built-in Python modules include os, sys, math, random, and so on. |
Popular built-in Python libraries include Pygame, Pytorch, matplotlib, and more. |
Conclusion
In this article, we learned about modules and libraries through examples. We also learned about the differences between modules and libraries.