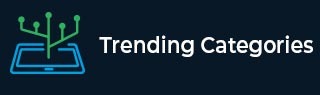
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
What does the pandas series.filter() method do?
The series.filter() method in the pandas series constructor is used to subset the rows of a series object based on the index labels. The filter method does not work for the content of the series object, it is only applied to the index labels of the series object.
The method won’t raise an error if the specified label does not match to the series index labels.
The parameters for the filter() method are items, like, regex, and axis. The items parameter takes a list-like object to access the set of rows from the given series object. The regex parameter is used to define a regular expression that can be used to retrieve the series rows.
Example 1
In this following example, we will filter out some rows in the pandas series object by specifying the list of index labels to the filter() method.
# importing pandas package import pandas as pd # creating pandas Series object series = pd.Series({'B':'black','Y':'yellow', 'W':'white','R':'red', 'Bl':'blue','G':'green','S':"silver", 'M':"maroon"}) print("Original Series:") print(series) print("Output: ") # Apply the filter method print(series.filter(['B','R','G','M']))
Explanation
Initially, we have created a series object using a python dictionary with pairs of keys and values. Here, the index labels are created by using keys of the dictionary.
Output
The output is given below −
Original Series: B black Y yellow W white R red Bl blue G green S silver M maroon dtype: object Output: B black R red G green M maroon dtype: object
We have successfully filtered the specified rows from the initial series object. And the resultant series object has the same data type as the input series object.
Example 2
Let’s take another series object to filter a single row using an index label and we must mention the single label value as an element of an iterable object otherwise, it will give an error.
# importing pandas package import pandas as pd # creating pandas Series object series = pd.Series({1:'East',2:'West',3:'North',4:'South',5:'East',6:'West',7:'North'}) print("Original Series:") print(series) print("Output: ") # Apply the filter method print(series.filter([2]))
Output
The output is given below −
Original Series: 1 East 2 West 3 North 4 South 5 East 6 West 7 North dtype: object Output: 2 West dtype: object
As we can see in the above output block, we have successfully filtered a single row label from the series object.