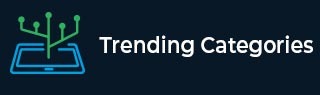
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
What do axes attribute in the pandas DataFrame?
The “axes” is an attribute of the pandas DataFrame, this attribute is used to access the group of rows and columns labels of the given DataFrame. It will return a python list representing the axes of the DataFrame.
The axes attribute collects all the row and column labels and returns a list object with all axes labels in it.
Example 1
In the following example, we initialized a DataFrame with some data. Then, we called the axes property on the DataFrame object.
# importing pandas package import pandas as pd # create a Pandas DataFrame df = pd.DataFrame([[1, 4, 3],[7, 2, 6],[6, 6, 2]], columns=['X', 'Y', 'Z']) print("DataFrame:") print(df) # apply .axes attribute result = df.axes print("Output:") print(result)
Output
The output is given below −
DataFrame: X Y Z 0 1 4 3 1 7 2 6 2 6 6 2 Output: [RangeIndex(start=0, stop=3, step=1), Index(['X', 'Y', 'Z'], dtype='object')]
The output for the axes attribute is a list which is holding the rows and columns labels of the DataFrame.
Example 2
In this example, we have initialized a DataFrame and we haven’t specified the index labels, so that the default index will be created. The .columns labels are assigned through keys of the python dictionary.
# importing pandas package import pandas as pd # create a Pandas DataFrame df = pd.DataFrame({'Col1':[1, 'q', 4], 'Col2':['a', 'w', 23]}) print("DataFrame:") print(df) # apply .axes attribute result = df.axes print("Output:") print(result)
Output
The output is given below −
DataFrame: Col1 Col2 0 1 a 1 q w 2 4 23 Output: [RangeIndex(start=0, stop=3, step=1), Index(['Col1', 'Col2'], dtype='object')]
We got the python list object as an output for the axes property and there are two elements present, one representing the row labels and the second one is holding the column names.