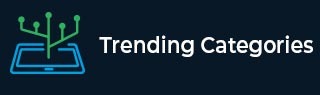
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
What are various Inheritance mapping strategies available in Hibernate?
There are three types of inheritance mapping strategies −
Table per class hierarchy
Table per concrete class
Table per subclass
In this article, we will discuss table per class hierarchy.
Table per class hierarchy
In this, only a single table is created for inheritance mapping. Disadvantages of this approach is that a lot of null values gets stored in the table.
@Inheritance(strategy=InheritanceType.SINGLE_TABLE), @DiscriminatorColumn and @DiscriminatorValue are the annotations used in this strategy.
@DiscriminatorColumn is used to create an additional column which is used to identify the hierarchy classes.
Consider the following example to understand this −
Steps to implement −
Create entity classes and use proper annotations for them.
Write the hibernate configuration file and add the mapping classes.
Write the code to create and store the data in the table.
1.CREATE ENTITY CLASSES
Car.java
package com.tutorialspoint; @Entity @Table(name = "car") @Inheritance(strategy=InheritanceType.SINGLE_TABLE) @DiscriminatorColumn(name="category",discriminatorType=DiscriminatorType.STRING) @DiscriminatorValue(value="car") public class Car { @Id @GeneratedValue(strategy=GenerationType.AUTO) private int id; @Column(name = "name") private String name; @Column(name = "color") private String color; //Getters //Setters }
Sports_Car.java
package com.tutorialspoint; import javax.persistence.*; @Entity @DiscriminatorValue("sportscar") public class Sports_Car extends Car{ @Column(name="mileage") private int mileage; @Column(name="cost") private int cost; //Getters //Setters }
Taxi_Car.java
package com.tutorialspoint; import javax.persistence.*; @Entity @DiscriminatorValue("taxicar") public class Taxi_Car extends Car{ @Column(name="farePerKm") private int farePerKm; @Column(name="available") private boolean available; //Getters //Setters }
2. Hibernate Configuration file (hibernate.cfg.xml)
<!DOCTYPE hibernate-configuration PUBLIC "-//Hibernate/Hibernate Configuration DTD 3.0//EN" "http://www.hibernate.org/dtd/hibernate-configuration-3.0.dtd"> <hibernate-configuration> <session-factory> <!-- JDBC Database connection settings --> <property name="connection.driver_class">com.mysql.cj.jdbc.Driver</property> <property name="connection.url">jdbc:mysql://localhost:3306/demo?useSSL=false</property> <property name="connection.username">root</property> <property name="connection.password">root</property> <!-- JDBC connection pool settings ... using built-in test pool --> <property name="connection.pool_size">4</property> <!-- Echo the SQL to stdout --> <property name="show_sql">true</property> <!-- Select our SQL dialect --> <property name="dialect">org.hibernate.dialect.MySQL5Dialect</property> <!-- Drop and re-create the database schema on startup --> <property name="hbm2ddl.auto">create-drop</property> <!-- name of annotated entity class --> <mapping class="com.tutorialspoint.Car"/> <mapping class="com.tutorialspoint.Sports_Car"/> <mapping class="com.tutorialspoint.Taxi_Car"/> </session-factory> </hibernate-configuration>
3. Code to create a table and store the data
package com.tutorialspoint; import org.hibernate.Session; import org.hibernate.SessionFactory; import org.hibernate.Transaction; import org.hibernate.cfg.Configuration; public class StoreTest { public static void main(String args[]){ SessionFactory sessionFactory = new Configuration() .configure("com/tutorialspoint/hibernate.cfg.xml") .buildSessionFactory(); Session session=sessionFactory.openSession(); Transaction t=session.beginTransaction(); Car c1=new Car(); c1.setName("Mercedes"); c1.setColor("Black"); Sport_Car c2=new Sport_Car(); c2.setName("Porsche"); c2.setColor("Red"); c2.setMileage(20); c2.setCost(5000000); Taxi_Car c3=new Taxi_Car(); c3.setName("Innova"); c3.setColor("White"); c3.setFarePerKm(7); c3.setAvailable(true); session.persist(c1); session.persist(c2); session.persist(c3); t.commit(); session.close(); } }