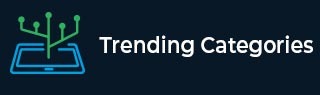
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
What are the rules for the body of lambda expression in Java?
A lambda expression is an anonymous function (nameless function) that has passed as an argument to another function. We need to follow some rules while using the body of a lambda expression.
Rules for the body of a lambda expression
- The body of the lambda expression can be either a single expression or more statements.
- If we are using a single expression as the body of a lambda expression, then no need to enclose the body with curly braces ({}).
- If we are using one or more statements as the body of a lambda expression, then enclosing them within curly braces({}) can be mandatory.
Syntax
(parameters) OR () -> {body with statements separated by;} OR Single Statement
Example
interface Message { String message(String name); } public class LambdaExpressionBodyTest { public static void main(String args[]) { // Lambda expression using single expressio Message msg1 = msg -> "TutorialsPoint " + msg; System.out.println(msg1.message("Lambda Expression With Expression")); // Lambda expression using statement Message msg2 = msg -> { return "TutorialsPoint " + msg; }; System.out.println(msg2.message("Lambda Expression With Statement")); // Lambda expression using multiple statements Message msg3 = msg -> { String hello = "TutorialsPoint " + msg; return hello; }; System.out.println(msg3.message("Lambda Expression With Multiple Statement")); } }
Output
TutorialsPoint Lambda Expression With Expression TutorialsPoint Lambda Expression With Statement TutorialsPoint Lambda Expression With Multiple Statement
Advertisements