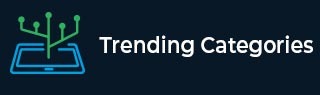
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
What are the differences between Widening Casting (Implicit) and Narrowing Casting (Explicit) in Java?
A Type casting in Java is used to convert objects or variables of one type into another. When we are converting or assigning one data type to another they might not compatible. If it is suitable then it will do smoothly otherwise chances of data loss.
Type Casting Types in Java
Java Type Casting is classified into two types.
- Widening Casting (Implicit) – Automatic Type Conversion
- Narrowing Casting (Explicit) – Need Explicit Conversion
Widening Casting (smaller to larger type)
Widening Type Conversion can happen if both types are compatible and the target type is larger than source type. Widening Casting takes place when two types are compatible and the target type is larger than the source type.
Example1
public class ImplicitCastingExample { public static void main(String args[]) { byte i = 40; // No casting needed for below conversion short j = i; int k = j; long l = k; float m = l; double n = m; System.out.println("byte value : "+i); System.out.println("short value : "+j); System.out.println("int value : "+k); System.out.println("long value : "+l); System.out.println("float value : "+m); System.out.println("double value : "+n); } }
Output
byte value : 40 short value : 40 int value : 40 long value : 40 float value : 40.0 double value : 40.0
Widening Casting of a Class Type
In the below example, Child class is the smaller type we are assigning it to Parent class type which is a larger type hence no casting is required.
Example2
class Parent { public void display() { System.out.println("Parent class display() called"); } } public class Child extends Parent { public static void main(String args[]) { Parent p = new Child(); p.display(); } }
Output
Parent class display() method called
Narrowing Casting (larger to smaller type)
When we are assigning a larger type to a smaller type, Explicit Casting is required.
Example1
public class ExplicitCastingExample { public static void main(String args[]) { double d = 30.0; // Explicit casting is needed for below conversion float f = (float) d; long l = (long) f; int i = (int) l; short s = (short) i; byte b = (byte) s; System.out.println("double value : "+d); System.out.println("float value : "+f); System.out.println("long value : "+l); System.out.println("int value : "+i); System.out.println("short value : "+s); System.out.println("byte value : "+b); } }
Output
double value : 30.0 float value : 30.0 long value : 30 int value : 30 short value : 30 byte value : 30
Narrowing a Class Type
When we are assigning larger type to a smaller type, then we need to explicitly typecast it.
Example2
class Parent { public void display() { System.out.println("Parent class display() method called"); } } public class Child extends Parent { public void display() { System.out.println("Child class display() method called"); } public static void main(String args[]) { Parent p = new Child(); Child c = (Child) p; c.display(); } }
Output
Child class display() method called