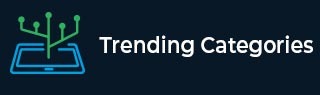
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
What are the differences between findElement() and findElements() methods?
findElement() and findElements() method tries to search an element in DOM.
The differences between them are listed below −
sl.no. | findElement() | findElements() |
---|---|---|
1 | It returns the first web element which matches with the locator. | It returns all the web elements which match with the locator. |
2 | Syntax − WebElement button = webdriver.findElement(By.name("<<Name value>>")); | Syntax − List<WebElement> buttons = webdriver.findElements(By.name("<<Name value>>")); |
3 | NoSuchElementException is thrown if there are no matching web elements | Empty list is returned if there are no matching elements. |
Example
Using findElements ().
import org.openqa.selenium.By; import org.openqa.selenium.Keys; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class RowFindElements { public static void main(String[] args) { System.setProperty("webdriver.chrome.driver", "C:\Users\ghs6kor\Desktop\Java\chromedriver.exe"); WebDriver driver = new ChromeDriver(); String url = "https://www.tutorialspoint.com/plsql/plsql_basic_syntax.htm"; driver.get(url); driver.manage().window().maximize(); driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS); // xpath with index appended to get the values from the row 1of table using findElements() , which returns a list List<WebElement> rows = driver.findElements(By.xpath("//table/tbody/tr[2]/td")); System.out.println(“The number of values in row 2 is “+ rows.size()); driver.close(); } }
Example
Using findElement ()
import org.openqa.selenium.By; import org.openqa.selenium.Keys; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class CssTagname { public static void main(String[] args) { System.setProperty("webdriver.chrome.driver", "C:\Users\ghs6kor\Desktop\Java\chromedriver.exe"); WebDriver driver = new ChromeDriver(); String url = "https://www.tutorialspoint.com/index.htm"; driver.get(url); driver.manage().timeouts().implicitlyWait(12, TimeUnit.SECONDS); //Using id tagname attribute combination for css expression //and get the element from findElement() driver.findElement(By.cssSelector("input[name=’search’]")). sendKeys("Selenium"); driver.close(); } }
Advertisements