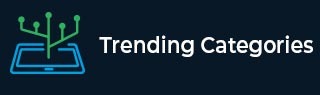
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
What are the differences between ClassNotFoundException and NoClassDefFoundError in Java?\n
Both ClassNotFoundException and NoClassDefFoundError are the errors when JVM or ClassLoader not able to find appropriate class while loading at run-time. ClassNotFoundException is a checked exception and NoClassDefFoundError is an Error which comes under unchecked.
There are different types of ClassLoader loads classes from difference sources, sometimes it may cause library JAR files missing or incorrect class-path which causes loader not able to load the class at run-time.
ClassNotFoundException
ClassNotFoundException comes when we try to load a class at run-time using Reflection and if those class files are missing then application or program thrown with ClassNotFoundException Exception. There is nothing to check at compile-time since it is loading the class at run-time.
Example
public class ClassNotFoundExceptionTest { public static void main(String[] args) { try { Class.forName("Test"); } catch (ClassNotFoundException cnfe) { System.err.println("You are trying to search for a class is not existing. "+cnfe); } } }
Output
You are trying to search for a class is not existing. java.lang.ClassNotFoundException: Test
NoClassDefFoundError
NoClassDefFoundError is thrown when a class has been compiled with a specific class from the classpath but if same class not available during run-time. Missing JAR files are the most basic reason to get NoClassDefFoundError. As per Java API docs "The searched-for class definition existed when the currently executing class was compiled, but the definition can no longer be found."
Example
class Test1 { public void show() { System.out.println("show() method called"); } } public class Test2 { public static void main(String[] args) { Test1 t = new Test1(); t.show(); } }
When we compile both the classes we will be getting two class files Test1.class and Test2.class, while running Test2 class just remove the Test1.class file then we will be getting NoClassDefFoundError as below
Output
Exception in thread "main" java.lang.NoClassDefFoundError: Test1 at Test2.main(Test2.java:9)